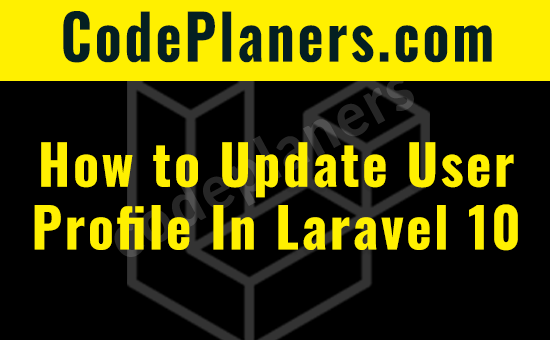
Hi Dev,
Today, we will show you how to update user profile in laravel 10. This article will give you simple example of how to update user profile in laravel 10. Let’s discuss how to update user profile in laravel 10. In this article, we will implement a how to update user profile in laravel 10.
So let’s follow few step to create example of how to update user profile in laravel 10.
- Step 1:Install Laravel 10
- Step 2: Setup Database Configuration
- Step 3: Install Auth
- Step 4: Create Migration
- Step 5: Create Route
- Step 6: Create Controller
- Step 7: Create Blade File
- Run Laravel 10
Step 1: Install Laravel 10
composer create-project laravel/laravel example-app
Step 2: Setup Database Configuration
.env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=Database_Name DB_USERNAME=Database_Username DB_PASSWORD=Database_Password
Step 3: Install Auth
composer require laravel/ui
Next
php artisan ui bootstrap --auth
install npm packages
npm install
Finally, the Bootstrap CSS was created using the command below:
npm run build
Step 4: Create Migration
php artisan make:migration add_new_fields_users
database/migrations/add_new_fields_users.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::table('users', function (Blueprint $table) { $table->string('avatar')->nullable(); $table->string('phone')->nullable(); $table->string('city')->nullable(); }); } /** * Reverse the migrations. */ public function down(): void { Schema::table('users', function (Blueprint $table) { $table->dropColumn(['avatar', 'phone', 'city']); }); } };
Now, run migration with following command
php artisan migrate
Next, update User.php model file
app/Models/User.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasApiTokens, HasFactory, Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', 'avatar', 'phone', 'city', ]; /** * The attributes that should be hidden for serialization. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', 'password' => 'hashed', ]; }
Step 5: Create Route
routes/web.php
<?php use Illuminate\Support\Facades\Route; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider and all of them will | be assigned to the "web" middleware group. Make something great! | */ Route::get('/', function () { return view('welcome'); }); Auth::routes(); Route::get('/home', [App\Http\Controllers\HomeController::class, 'index'])->name('home'); Route::get('/profile', [App\Http\Controllers\ProfileController::class, 'index'])->name('user.profile'); Route::post('/profile', [App\Http\Controllers\ProfileController::class, 'store'])->name('user.profile.store');
Step 6: Create Controller
app/Http/Controllers/ProfileController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Hash; class ProfileController extends Controller { /** * Create a new controller instance. * * @return void */ public function __construct() { $this->middleware('auth'); } /** * Show the application dashboard. * * @return \Illuminate\Contracts\Support\Renderable */ public function index() { return view('profile'); } /** * Write code on Method * * @return response() */ public function store(Request $request) { $request->validate([ 'name' => 'required', 'email' => 'required', 'confirm_password' => 'required_with:password|same:password', 'avatar' => 'image', ]); $input = $request->all(); if ($request->hasFile('avatar')) { $avatarName = time().'.'.$request->avatar->getClientOriginalExtension(); $request->avatar->move(public_path('avatars'), $avatarName); $input['avatar'] = $avatarName; } else { unset($input['avatar']); } if ($request->filled('password')) { $input['password'] = Hash::make($input['password']); } else { unset($input['password']); } auth()->user()->update($input); return back()->with('success', 'Profile updated successfully.'); } }
Step 7: Create Blade File
resources/views/profile.blade.php
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-12"> <div class="card"> <div class="card-header">{{ __('Profile') }}</div> <div class="card-body"> <form method="POST" action="{{ route('user.profile.store') }}" enctype="multipart/form-data"> @csrf @if (session('success')) <div class="alert alert-success" role="alert" class="text-danger"> {{ session('success') }} </div> @endif <div class="row"> <div class="mb-3 col-md-6"> <label for="name" class="form-label">Avatar: </label> <input id="avatar" type="file" class="form-control @error('avatar') is-invalid @enderror" name="avatar" value="{{ old('avatar') }}" autocomplete="avatar"> @error('avatar') <span role="alert" class="text-danger"> <strong>{{ $message }}</strong> </span> @enderror </div> <div class="mb-3 col-md-6"> <img src="/avatars/{{ auth()->user()->avatar }}" style="width:80px;margin-top: 10px;"> </div> </div> <div class="row"> <div class="mb-3 col-md-6"> <label for="name" class="form-label">Name: </label> <input class="form-control" type="text" id="name" name="name" value="{{ auth()->user()->name }}" autofocus="" > @error('name') <span role="alert" class="text-danger"> <strong>{{ $message }}</strong> </span> @enderror </div> <div class="mb-3 col-md-6"> <label for="email" class="form-label">Email: </label> <input class="form-control" type="text" id="email" name="email" value="{{ auth()->user()->email }}" autofocus="" > @error('email') <span role="alert" class="text-danger"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row"> <div class="mb-3 col-md-6"> <label for="password" class="form-label">Password: </label> <input class="form-control" type="password" id="password" name="password" autofocus="" > @error('password') <span role="alert" class="text-danger"> <strong>{{ $message }}</strong> </span> @enderror </div> <div class="mb-3 col-md-6"> <label for="confirm_password" class="form-label">Confirm Password: </label> <input class="form-control" type="password" id="confirm_password" name="confirm_password" autofocus="" > @error('confirm_password') <span role="alert" class="text-danger"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row"> <div class="mb-3 col-md-6"> <label for="phone" class="form-label">Phone: </label> <input class="form-control" type="text" id="phone" name="phone" value="{{ auth()->user()->phone }}" autofocus="" > @error('phone') <span role="alert" class="text-danger"> <strong>{{ $message }}</strong> </span> @enderror </div> <div class="mb-3 col-md-6"> <label for="city" class="form-label">City: </label> <input class="form-control" type="text" id="city" name="city" value="{{ auth()->user()->city }}" autofocus="" > @error('city') <span role="alert" class="text-danger"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-0"> <div class="col-md-12 offset-md-5"> <button type="submit" class="btn btn-primary"> {{ __('Upload Profile') }} </button> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
resources/views/layouts/app.blade.php
<!-- Right Side Of Navbar --> <ul class="navbar-nav ms-auto"> <!-- Authentication Links --> @guest @if (Route::has('login')) <li class="nav-item"> <a class="nav-link" href="{{ route('login') }}">{{ __('Login') }}</a> </li> @endif @if (Route::has('register')) <li class="nav-item"> <a class="nav-link" href="{{ route('register') }}">{{ __('Register') }}</a> </li> @endif @else <li class="nav-item dropdown"> <a id="navbarDropdown" class="nav-link dropdown-toggle" href="#" role="button" data-bs-toggle="dropdown" aria-haspopup="true" aria-expanded="false" v-pre> <img src="/avatars/{{ Auth::user()->avatar }}" style="width: 30px; border-radius: 10%"> {{ Auth::user()->name }} </a> <div class="dropdown-menu dropdown-menu-end" aria-labelledby="navbarDropdown"> <a href="{{ route('user.profile') }}" class="dropdown-item">Profile</a> <a class="dropdown-item" href="{{ route('logout') }}" onclick="event.preventDefault(); document.getElementById('logout-form').submit();"> {{ __('Logout') }} </a> <form id="logout-form" action="{{ route('logout') }}" method="POST" class="d-none"> @csrf </form> </div> </li> @endguest </ul>
Run Laravel
php artisan serve
I hope it will assist you…