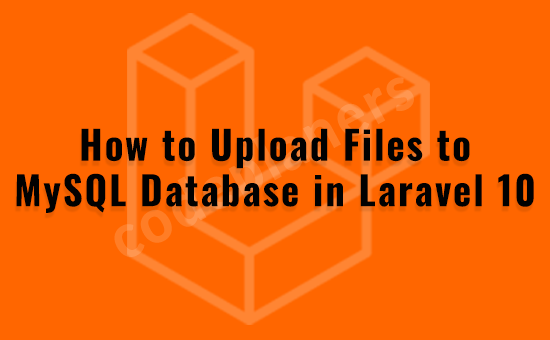
Hi dev,
Today, i show you how to upload files to MySQL database in laravel 10. In this article will tell you how to upload files to MySQL database in laravel 10. you will how to upload files to MySQL database in laravel 10.
So, let’s follow few steps to create example of how to upload files to MySQL database in laravel 10.
Step 1: Install Laravel
composer create-project laravel/laravel example-blog
Step 2: Create Table and Model
php artisan create_files_table
database/migrations/migration_file.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; use Illuminate\Support\Facades\DB; return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('files', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('mime'); $table->timestamps(); }); DB::statement("ALTER TABLE files ADD file MEDIUMBLOB"); } /** * Reverse the migrations. */ public function down(): void { Schema::dropIfExists('files'); } };
Step 3: Create Controller
php artisan make:controller FileController
app/Http/Controllers/FileController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\View\View; use Illuminate\Http\RedirectResponse; use App\Models\File; class FileController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(): View { $files = File::latest()->get(); return view('fileUpload', compact('files')); } /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function store(Request $request): RedirectResponse { $request->validate([ 'file' => 'mimes:jpeg,bmp,png,pdf,jpg', 'name' => 'required|max:255', ]); if ($request->hasFile('file')) { $path = $request->file('file')->getRealPath(); $ext = $request->file->extension(); $doc = file_get_contents($path); $base64 = base64_encode($doc); $mime = $request->file('file')->getClientMimeType(); File::create([ 'name'=> $request->name .'.'.$ext, 'file' => $base64, 'mime'=> $mime, ]); } return back() ->with('success','You have successfully upload file.'); } }
Step 4: Create Add Routes
routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\FileController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('file-upload', [FileController::class, 'index']); Route::post('file-upload', [FileController::class, 'store'])->name('file.store');
Step 5: Create Blade File
resources/views/fileUpload.blade.php
<!DOCTYPE html> <html> <head> <title>How to Upload Files to MySQL Database in Laravel 10 - codeplaners.com</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"> </head> <body> <div class="container"> <div class="panel panel-primary"> <div class="panel-heading"> <h2>How to Upload Files to MySQL Database in Laravel 10 - codeplaners.com</h2> </div> <div class="panel-body"> @if ($message = Session::get('success')) <div class="alert alert-success alert-block"> <strong>{{ $message }}</strong> </div> @endif <div class="row"> @if ($files->count()) <h3>Files</h3> <table class="table table-striped table-bordered table-hover"> <thead> <tr> <td>Name</td> <td></td> </tr> </thead> <tbody> @foreach ($files as $file) <tr> <td>{{ $file->name }}</td> <td><img src="data:{{ $file->mime }};base64,{{ $file->file }}" style="height: 100px; width: auto"></td> </tr> </tbody> @endforeach </table> @endif </div> <h3>Upload File:</h3> <form action="{{ route('file.store') }}" method="POST" enctype="multipart/form-data"> @csrf <div class="mb-3"> <label class="form-label" for="inputFile">Name:</label> <input type="text" name="name" id="inputFile" class="form-control @error('name') is-invalid @enderror"> @error('name') <span class="text-danger">{{ $message }}</span> @enderror </div> <div class="mb-3"> <label class="form-label" for="inputFile">File:</label> <input type="file" name="file" id="inputFile" class="form-control @error('file') is-invalid @enderror"> @error('file') <span class="text-danger">{{ $message }}</span> @enderror </div> <div class="mb-3"> <button type="submit" class="btn btn-success">Upload</button> </div> </form> </div> </div> </div> </body> </html>
Run Laravel App:
php artisan serve
I hope it will assist you…