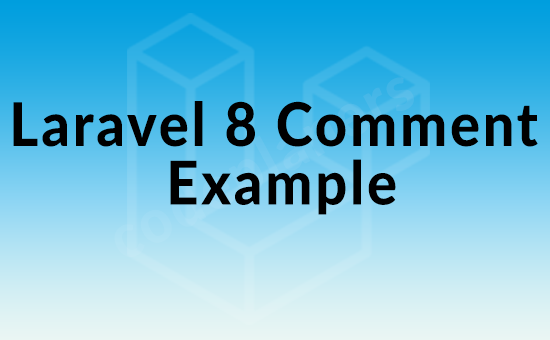
This post was last updated on May 15th, 2021 at 11:25 pm
Today, i we will show you laravel 8 comment example. This article will give you simple example of laravel 8 comment example. you will learn laravel 8 comment example. step by step explain laravel 8 comment example.
we will create very simple comment example with you can add comment and make reply to comment. we will use laravel 8 relationship for comment example and it make it quick.
Step 1 :- Install Laravel 8
composer create-project --prefer-dist laravel/laravel comment
Connect Database .env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=comment DB_USERNAME=root DB_PASSWORD=
Step 2:- Create Post and Comment Table
php artisan make:migration create_posts_comments_table
database/migrationscreate_posts_comments_table.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreatePostsCommentsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('posts', function (Blueprint $table) { $table->increments('id'); $table->string('title'); $table->text('body'); $table->timestamps(); $table->softDeletes(); }); Schema::create('comments', function (Blueprint $table) { $table->increments('id'); $table->integer('user_id')->unsigned(); $table->integer('post_id')->unsigned(); $table->integer('parent_id')->unsigned()->nullable(); $table->text('body'); $table->timestamps(); $table->softDeletes(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('posts'); Schema::dropIfExists('comments'); } }
Now run this below command to migrate our post and comment table
php artisan migrate
Step 3:- Create Auth
You need to follow some few steps to complete auth in your laravel 8 application. First you need to install laravel/ui package.
composer require laravel/ui
following commands for creating auth
php artisan ui vue --auth
Step 4:- Create Model and Relationship
In this step, we need to add model Post and Comment for table. we also need to make code for laravel relationship for comments.
php artisan make:model Post
app/Models/Post.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class Post extends Model { use HasFactory, SoftDeletes; protected $dates = ['deleted_at']; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = ['title', 'body']; /** * The has Many Relationship * * @var array */ public function comments() { return $this->hasMany(Comment::class)->whereNull('parent_id'); } }
php artisan make:model Comment
app/Models/Comment.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class Comment extends Model { use HasFactory, SoftDeletes; protected $dates = ['deleted_at']; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = ['user_id', 'post_id', 'parent_id', 'body']; /** * The belongs to Relationship * * @var array */ public function user() { return $this->belongsTo(User::class); } /** * The has Many Relationship * * @var array */ public function replies() { return $this->hasMany(Comment::class, 'parent_id'); } }
Step 5:- Create Controller
In this step, now we should add new controller as PostController and CommentController. So run bellow command and create new controller.
Create Post Controller using bellow command
php artisan make:controller PostController
app/Http/Controllers/PostController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Post; class PostController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $posts = Post::all(); return view('posts.index', compact('posts')); } /** * Show the form for creating a new resource. * * @return \Illuminate\Http\Response */ public function create() { return view('posts.create'); } /** * Store a newly created resource in storage. * * @param \Illuminate\Http\Request $request * @return \Illuminate\Http\Response */ public function store(Request $request) { $request->validate([ 'title'=>'required', 'body'=>'required', ]); Post::create($request->all()); return redirect('/posts'); } /** * Show the form for creating a new resource. * * @return \Illuminate\Http\Response */ public function show($id) { $post = Post::find($id); return view('posts.show', compact('post')); } }
Create Comment Controller using bellow command
php artisan make:controller CommentController
app/Http/Controllers/CommentController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Comment; use App\Models\Post; class CommentController extends Controller { /** * Store a newly created resource in storage. * * @param \Illuminate\Http\Request $request * @return \Illuminate\Http\Response */ public function store(Request $request) { $request->validate([ 'body'=>'required', ]); $input = $request->all(); $input['user_id'] = auth()->user()->id; Comment::create($input); return back(); } }
Step 6:- Create Routes
<?php use Illuminate\Support\Facades\Route; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('/', function () { return view('welcome'); }); Auth::routes(); Route::get('/home', [App\Http\Controllers\HomeController::class, 'index'])->name('home'); Route::get('/post', [App\Http\Controllers\PostController::class, 'create', ])->name('post.create'); Route::post('/post', [App\Http\Controllers\PostController::class, 'store'])->name('post.store'); Route::get('/posts', [App\Http\Controllers\PostController::class, 'index'])->name('posts'); Route::get('/article/{post:slug}', [App\Http\Controllers\PostController::class, 'show'])->name('post.show'); Route::post('/comment/store', [App\Http\Controllers\CommentController::class, 'store'])->name('comment.add');
Step 7:- Create Blade Files
In last step. In this step we have to add blade files. So we have to add ‘posts’ folder and in the posts folder we will create our view file.
1:- index.blade.php
2:- show.blade.php
3:- create.blade.php
4:- commentsDisplay.blade.php
resources/views/posts/index.blade.php
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-12"> <h1>Manage Posts</h1> <a href="{{ url('/post') }}" class="btn btn-success" style="float: right">Create Post</a> <table class="table table-bordered"> <thead> <th width="80px">Id</th> <th>Title</th> <th width="150px">Action</th> </thead> <tbody> @foreach($posts as $post) <tr> <td>{{ $post->id }}</td> <td>{{ $post->title }}</td> <td> <a href="{{ url('article', $post->id) }}" class="btn btn-primary">View Post</a> </td> </tr> @endforeach </tbody> </table> </div> </div> </div> @endsection
resources/views/posts/show.blade.php
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-body"> <h3 class="text-center text-success">Codeplaners.com</h3> <br/> <h2>{{ $post->title }}</h2> <p> {{ $post->body }} </p> <hr /> <h4>Display Comments</h4> @include('posts.commentsDisplay', ['comments' => $post->comments, 'post_id' => $post->id]) <hr /> <h4>Add comment</h4> <form method="post" action="{{ url('/comment/store' ) }}"> @csrf <div class="form-group"> <textarea class="form-control" name="body"></textarea> <input type="hidden" name="post_id" value="{{ $post->id }}" /> </div> <div class="form-group"> <input type="submit" class="btn btn-success" value="Add Comment" /> </div> </form> </div> </div> </div> </div> </div> @endsection
resources/views/posts/create.blade.php
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">Create Post</div> <div class="card-body"> <form method="post" action="{{ url('/post') }}"> <div class="form-group"> @csrf <label class="label">Post Title: </label> <input type="text" name="title" class="form-control" required/> </div> <div class="form-group"> <label class="label">Post Body: </label> <textarea name="body" rows="10" cols="30" class="form-control" required></textarea> </div> <div class="form-group"> <input type="submit" class="btn btn-success" /> </div> </form> </div> </div> </div> </div> </div> @endsection
resources/views/posts/commentsDisplay.blade.php
@foreach($comments as $comment) <div class="display-comment" @if($comment->parent_id != null) style="margin-left:40px;" @endif> <strong>{{ $comment->user->name }}</strong> <p>{{ $comment->body }}</p> <a href="" id="reply"></a> <form method="post" action="{{ url('/comment/store' ) }}"> @csrf <div class="form-group"> <input type="text" name="body" class="form-control" /> <input type="hidden" name="post_id" value="{{ $post_id }}" /> <input type="hidden" name="parent_id" value="{{ $comment->id }}" /> </div> <div class="form-group"> <input type="submit" class="btn btn-warning" value="Reply" /> </div> </form> @include('posts.commentsDisplay', ['comments' => $comment->replies]) </div> @endforeach
Now we are ready to run command
php artisan serve
Now you can open bellow URL on your browser
http://127.0.0.1:8000/posts