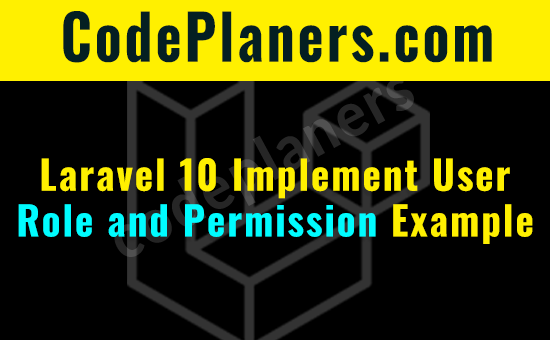
This post was last updated on January 1st, 2025 at 06:38 am
Hi Dev,
Today, we will show you laravel 10 implement user role and permission example. This article will give you simple example of laravel 10 implement user role and permission example. Let’s discuss how to set role permissions. In this article, we will implement a laravel 10 implement user role and permission example.
So let’s follow few step to create example of laravel 10 implement user role and permission example.
Install the Spatie Permission package.
composer require spatie/laravel-permission
Publish the package’s configuration and migration files.
php artisan vendor:publish --provider="Spatie\Permission\PermissionServiceProvider"
Migrate the database.
php artisan migrate
Create the Role and Permission models.
php artisan make:model Role -m php artisan make:model Permission -m
Update User Model File
class User extends Model { use HasFactory, SoftDeletes; protected $fillable = [ 'name', 'email', 'password', ]; protected $hidden = [ 'password', 'remember_token', ]; protected $casts = [ 'email_verified_at' => 'datetime', ]; public function roles() { return $this->belongsToMany(Role::class); } public function permissions() { return $this->belongsToMany(Permission::class); } }
Create Role and Permission seeders
php artisan make:seeder RoleSeeder php artisan make:seeder PermissionSeeder
RoleSeeder create some roles and add them to the database
class RoleSeeder extends Seeder { public function run() { $adminRole = Role::create(['name' => 'admin']); $userRole = Role::create(['name' => 'user']); $adminRole->givePermissionTo('all'); } }
PermissionSeeder create certain permissions and then seed them into the database.
class PermissionSeeder extends Seeder { public function run() { Permission::create(['name' => 'view users']); Permission::create(['name' => 'create users']); Permission::create(['name' => 'edit users']); Permission::create(['name' => 'delete users']); } }
Run the seeders
php artisan db:seed
Assign roles to users based on their responsibilities or access levels.
$user = User::find(1); $user->assignRole('admin');
Verify whether the user has a specific role or permission.
$user = User::find(1); if ($user->hasRole('admin')) { // do something } if ($user->can('view users')) { // do something }
You can also use middleware for security based on user roles. For example, the following middleware will allow access to the /users root exclusively for users with the ‘admin’ role.
Route::middleware('role:admin')->get('/users', function () { // ... });
I hope it will assist you…