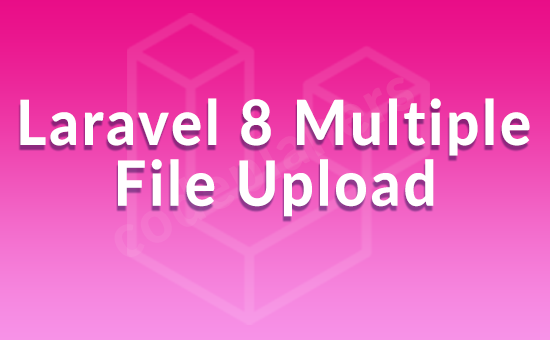
Today, we will show you how to upload multiple image in laravel 8. This article will give you simple example of how to upload multiple image in laravel 8. you will learn how to upload multiple image in laravel 8.
Now follow step by step. It is very simple. You can also upload file using this code not only image. So you can use this procedure to upload image or file. Let’s start.
Step 1 :- Install Laravel
composer create-project --prefer-dist laravel/laravel blog
Step 2 :- Create migration
php artisan make:model Image -m -r
database/migrations/images.php
use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateImageTable extends Migration { public function up() { Schema::create('images', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('image'); $table->timestamps(); }); } public function down() { Schema::dropIfExists('users'); } }
php artisan migrate
Image model
namespace App; use Illuminate\Database\Eloquent\Model; class Image extends Model { protected $guarded = []; }
Step 3 :- Route
routes/web.php
Route::resource('image','ImageController');
Now we have to create a trait. If you don’t know what is trait in php then please read this article before.
What is Traits in PHP ?
Step 4 :- Create a Trait
create fileApp/Traits/ImageUpload.php
namespace App\Traits; use Illuminate\Http\UploadedFile; use Illuminate\Support\Facades\Storage; trait ImageUpload { public function UserImageUpload($query) // Taking input image as parameter { $image_name = str_random(20); $ext = strtolower($query->getClientOriginalExtension()); // You can use also getClientOriginalName() $image_full_name = $image_name.'.'.$ext; $upload_path = 'image/'; //Creating Sub directory in Public folder to put image $image_url = $upload_path.$image_full_name; $success = $query->move($upload_path,$image_full_name); return $image_url; // Just return image } }
Step 5 :- ImageController
namespace App\Http\Controllers\ImageController use App\Http\Controllers\Controller; use App\Image; use App\Traits\ImageUpload; use Illuminate\Http\Request; class ImageController extends Controller{ use ImageUpload; //Using our created Trait to access in trait method public function store(Request $request) { $request->validate([ 'image' => 'required' ]); if ($request->hasFile('image')) { foreach($request->file('image ') as $file){ $filePath = $this->UserImageUpload($file); //passing parameter to our trait method one after another using foreach loop Image::create([ 'name' => $filePath, ]); } } return redirect()->back(); } }
Step 6 :- Create our blade file
<form action="{{ route('image.store') }}" method="post" enctype="multipart/form-data"> @csrf <input type="file" name="image[]" required="" multiple > <input type="submit" value="submit"> </form>