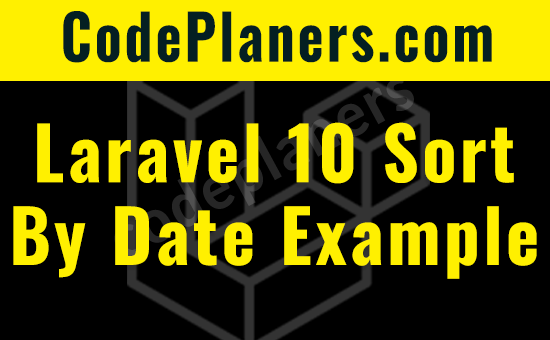
This post was last updated on January 1st, 2025 at 06:40 am
Hi dev,
Today, i show you laravel 10 sort by date example. In this article will tell you laravel 10 sort by date example. you will laravel 10 sort by date example.
We will use sortBy() method to sort by date in Laravel 10. I will give you simple two examples with output so that you can understand from tick.
So, let’s follow few steps to create example of laravel 10 sort by date example.
Example 1:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class UserController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(Request $request) { $collection = collect([ ['id' => 1, 'name' => 'codeplaners', 'created_at' => '2023-11-07'], ['id' => 2, 'name' => 'mohan', 'created_at' => '2023-11-08'], ['id' => 3, 'name' => 'brijesh', 'created_at' => '2023-11-05'], ['id' => 4, 'name' => 'krishan', 'created_at' => '2023-11-04'], ]); $sorted = $collection->sortBy('created_at'); $sorted = $sorted->all(); dd($sorted); } }
Output:
Array ( [3] => Array ( [id] => 4 [name] => krishan [created_at] => 2023-11-04 ) [2] => Array ( [id] => 3 [name] => brijesh [created_at] => 2023-11-05 ) [0] => Array ( [id] => 1 [name] => codeplaners [created_at] => 2023-11-07 ) [1] => Array ( [id] => 2 [name] => mohan [created_at] => 2023-11-08 ) )
Example 2:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class UserController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(Request $request) { $collection = collect(['2023-11-07', '2023-11-08', '2023-11-05', '2023-11-04']); $sorted = $collection->sortBy(function ($date) { return \Carbon\Carbon::createFromFormat('Y-m-d', $date); }); $sorted = $sorted->all(); dd($sorted); } }
Output:
Array ( [3] => 2023-11-04 [2] => 2023-11-05 [0] => 2023-11-07 [1] => 2023-11-08 )
I hope it will assist you…