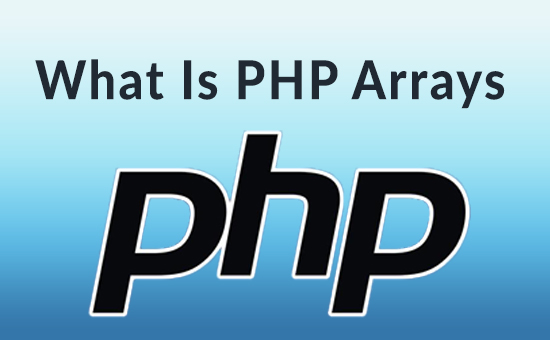
Arrays in PHP area unit a kind of information structure. that permits us to store multiple components of a similar knowledge sort underneath one variable, which saves us from making an attempt to make a separate variable for every knowledge.
In PHP primarily arrays of 3 types:
Indexed or Numeric Arrays: AN array with a numeric index wherever values area unit hold on linearly.
Associative Arrays: AN array with a string index wherever rather than linear storage, every price will be allotted a selected key.
Multidimensional Arrays: AN array that contains single or multiple arrays among it and might be accessed via multiple indices.
Indexed or Numeric Arrays
These arrays will store numbers, strings and any object however their index are described by numbers. By default array index starts from zero. These arrays is created in 2 alternative ways as shown within the following example:
<!doctype html> <html> <body> <?php /* First method to create array. */ $numbers = array( 1, 2, 3, 4, 5); foreach( $numbers as $value ) { echo "Value is $value <br />"; } /* Second method to create array. */ $numbers[0] = "one"; $numbers[1] = "two"; $numbers[2] = "three"; $numbers[3] = "four"; $numbers[4] = "five"; foreach( $numbers as $value ) { echo "Value is $value <br />"; } ?> </body> </html>
Result:-
Value is 1 Value is 2 Value is 3 Value is 4 Value is 5 Value is one Value is two Value is three Value is four Value is five
Associative Arrays
These sorts of arrays square measure like the indexed arrays however rather than linear storage, each price is allotted with a user-defined key of string sort.
NOTE:− do not keep associative array within double quote whereas printing otherwise it might not come back any price.
<!doctype html> <html> <body> <?php /* First method to associate create array. */ $salaries = array("anthony" => 2000, "ram" => 1000, "zara" => 500); echo "Salary of anthony is ". $salaries['anthony'] . "<br />"; echo "Salary of ram is ". $salaries['ram']. "<br />"; echo "Salary of zara is ". $salaries['zara']. "<br />"; /* Second method to create array. */ $salaries['anthony'] = "high"; $salaries['ram'] = "medium"; $salaries['zara'] = "low"; echo "Salary of anthony is ". $salaries['anthony'] . "<br />"; echo "Salary of ram is ". $salaries['ram']. "<br />"; echo "Salary of zara is ". $salaries['zara']. "<br />"; ?> </body> </html>
Result:-
Salary of anthony is 2000 Salary of ram is 1000 Salary of zara is 500 Salary of anthony is high Salary of ram is medium Salary of zara is low
Multidimensional Arrays
Multi-dimensional arrays area unit such arrays that store another array at every index rather than one part. In alternative words, we will outline multi-dimensional arrays as associate array of arrays. because the name suggests, each part during this array will be associate array and that they can even hold alternative sub-arrays at intervals. Arrays or sub-arrays in three-d arrays will be accessed victimization multiple dimensions.
Example:
<!doctype html> <html> <body> <?php // Add a multidimensional array $favorites = array( array( "name" => "Ram", "mob" => "6489715523", "email" => "ram@gmail.com", ), array( "name" => "Anthony", "mob" => "9884358721", "email" => "anthony@gmail.com", ), array( "name" => "Zara", "mob" => "9829601023", "email" => "zara@gmail.com", ) ); // Accessing elements echo "Ram E-mail is: " . $favorites[0]["email"], "\n"; echo "Zara mobile number is: " . $favorites[2]["mob"]; ?> </body> </html>
Result:-
Ram E-mail is: ram@gmail.com Zara mobile number is: 9829601023