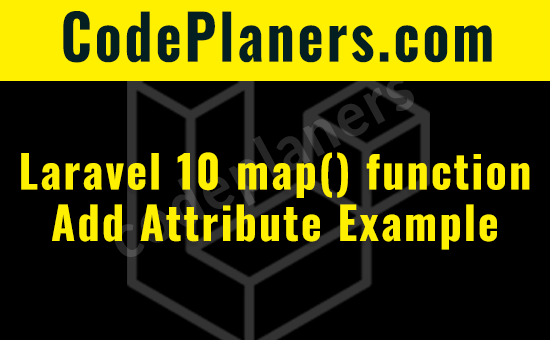
This post was last updated on January 1st, 2025 at 06:40 am
Hi dev,
Today, i show you laravel 10 map() function add attribute example. In this article will tell you laravel 10 map() function add attribute example. you will laravel 10 map() function add attribute example.
You can also use this example with Laravel 6, Laravel 7, Laravel 8, Laravel 9 and Laravel 10 versions.
If we are posting with status and you need to set label on status like “0” means “Inactive” and “1” means “Active”, then you can do it using laravel collection map() function.
So, let’s follow few steps to create example of laravel 10 map() function add attribute example.
Example 1: setAttribute()
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $user = User::first(); $user->setAttribute('position', 'Web Developer'); dd($user->toArray()); } }
Output:
array:8 [ "id" => 1 "name" => "Mohan" "email" => "codeplaners@gmail.com" "email_verified_at" => null "created_at" => "2022-06-23T07:02:17.000000Z" "updated_at" => "2022-06-23T07:02:17.000000Z" "birthdate" => "1998-11-15" "position" => "Web Developer" ]
Example 2: map()
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Str; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $posts = collect([ ['id'=>1,'title'=>'Post One'], ['id'=>2,'title'=>'Post Two'], ['id'=>3,'title'=>'Post Three'], ['id'=>4,'title'=>'Post Four'], ]); $posts = $posts->map(function ($post) { $post['url'] = url("post/". Str::slug($post['title'])); return $post; }); dd($posts->toArray()); } }
Output:
Array ( [0] => Array ( [id] => 1 [title] => Post One [url] => http://localhost:8000/post/post-one ) [1] => Array ( [id] => 2 [title] => Post Two [url] => http://localhost:8000/post/post-two ) [2] => Array ( [id] => 3 [title] => Post Three [url] => http://localhost:8000/post/post-three ) [3] => Array ( [id] => 4 [title] => Post Four [url] => http://localhost:8000/post/post-four ) )
Example 3: Laravel 10 Eloquent Collection Add Attribute using map()
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Post; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $posts = Post::select("id", "title", "status")->take(5)->get(); $posts = $posts->map(function ($post) { $post->status_label = $post->status == 1 ? 'Active' : 'InActive'; return $post; }); dd($posts->toArray()); } }
Output:
Array ( [0] => Array ( [id] => 2 [title] => Demo Updated [status] => 1 [status_label] => Active ) [1] => Array ( [id] => 3 [title] => Dr. [status] => 0 [status_label] => InActive ) [2] => Array ( [id] => 4 [title] => Miss [status] => 0 [status_label] => InActive ) [3] => Array ( [id] => 5 [title] => Dr. [status] => 0 [status_label] => InActive ) [4] => Array ( [id] => 6 [title] => Mrs. [status] => 0 [status_label] => InActive ) )
I hope it will assist you…