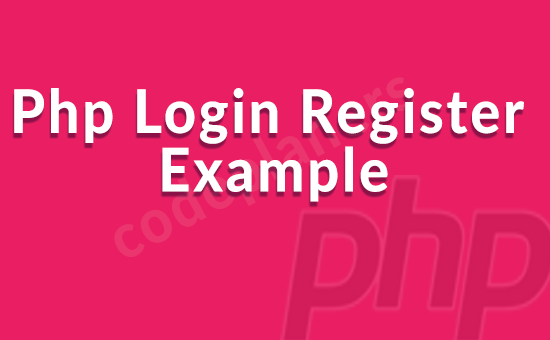
Today, i we will show you Php Login Register Example. This article will give you simple example of Php Login Register Example. you will learn Php Login Register Example. step by step explain Php Login Register Example.
Step 1:- Create Login Design Form
<?php include('server.php') ?> <!DOCTYPE html> <html> <head> <title>Php Login Register Example</title> <!-- -------------custum css link---------------- --> <link rel="stylesheet" type="text/css" href="style.css"> </head><!-- --------------head --> <body><!-- --------------body --> <div class="header"> <h2>Login</h2> </div><!-- -------------header --> <form method="post" action="login.php"> <?php if (count($errors) > 0) : ?> <div class="error"> <?php foreach ($errors as $error) : ?> <p><?php echo $error ?></p> <?php endforeach ?> </div> <?php endif ?> <div class="input-group"> <label>Username</label> <input type="text" name="username" > </div> <div class="input-group"> <label>Password</label> <input type="password" name="password"> </div> <div class="input-group"> <button type="submit" class="btn" name="login_user">Login</button> </div> <p> Not yet a member? <a href="register.php">Sign up</a> </p> </form><!-- -------------form --> </body> </html>
Step 2:- Create Register Design Form
<?php include('server.php') ?> <!DOCTYPE html> <html><head> <title>Php Login Register Example</title> <!-- -------------custum css link---------------- --> <link rel="stylesheet" type="text/css" href="style.css"> </head><!-- --------------head --> <body><!-- --------------body --> <div class="header"> <h2>Register</h2> </div><!-- ------------header --> <form method="post" action="register.php"> <?php if (count($errors) > 0) : ?> <div class="error"> <?php foreach ($errors as $error) : ?> <p><?php echo $error ?></p> <?php endforeach ?> </div> <?php endif ?> <div class="input-group"> <label>Username</label> <input type="text" name="username" value="<?php echo $username; ?>"> </div> <div class="input-group"> <label>Email</label> <input type="email" name="email" value="<?php echo $email; ?>"> </div> <div class="input-group"> <label>Password</label> <input type="password" name="password_1"> </div> <div class="input-group"> <label>Confirm password</label> <input type="password" name="password_2"> </div> <div class="input-group"> <button type="submit" class="btn" name="reg_user">Register</button> </div> <p> Already a member? <a href="login.php">Sign in</a> </p> </form><!-- ------------------form --> </body> </html>
Step 3:- Create the Database and setting up Tables
CREATE TABLE IF NOT EXISTS `accounts` ( `id` int(11) NOT NULL AUTO_INCREMENT, `username` varchar(50) NOT NULL, `password` varchar(255) NOT NULL, `email` varchar(100) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8; INSERT INTO `accounts` (`id`, `username`, `password`, `email`) VALUES (1, 'codeplaners', '$2y$10$SfhYIDtn.iOuCW7zfoFLuuZHX6lja4lF4XA4JqNmpiH/.P3zB8JCa', 'codeplaners@gmail.com');
Step 4:- Authenticating Users with PHP
<?php session_start(); $username = ""; $email = ""; $errors = array(); // database $db = mysqli_connect('localhost', 'root', '', 'registration_php'); // REGISTER if (isset($_POST['reg_user'])) { $username = mysqli_real_escape_string($db, $_POST['username']); $email = mysqli_real_escape_string($db, $_POST['email']); $password_1 = mysqli_real_escape_string($db, $_POST['password_1']); $password_2 = mysqli_real_escape_string($db, $_POST['password_2']); if (empty($username)) { array_push($errors, "Username is required"); } if (empty($email)) { array_push($errors, "Email is required"); } if (empty($password_1)) { array_push($errors, "Password is required"); } if ($password_1 != $password_2) { array_push($errors, "The two passwords do not match"); } $user_check_query = "SELECT * FROM accounts WHERE username='$username' OR email='$email' LIMIT 1"; $result = mysqli_query($db, $user_check_query); $user = mysqli_fetch_assoc($result); if ($user) { if ($user['username'] === $username) { array_push($errors, "Username already exists"); } if ($user['email'] === $email) { array_push($errors, "email already exists"); } } if (count($errors) == 0) { $password = md5($password_1); $query = "INSERT INTO accounts (username, email, password) VALUES('$username', '$email', '$password')"; mysqli_query($db, $query); $_SESSION['username'] = $username; $_SESSION['success'] = "You are now logged in"; header('location: index.php'); // echo "register successfully"; } } //---------------------------------- LOGIN----------------------------/// //-----------------------------------++++++---------------------------/// //----------------------------------login----------------------------//// if (isset($_POST['login_user'])) { $username = mysqli_real_escape_string($db, $_POST['username']); $password = mysqli_real_escape_string($db, $_POST['password']); // print_r($username); // print_r($password); // die(); if (empty($username)) { array_push($errors, "Username is required"); } if (empty($password)) { array_push($errors, "Password is required"); } if (count($errors) == 0) { $password = md5($password); $query = "SELECT * FROM accounts WHERE username='$username' AND password='$password'"; $results = mysqli_query($db, $query); // print_r($results); // die(); if (mysqli_num_rows($results) == 1) { $_SESSION['username'] = $username; $_SESSION['success'] = "You are now logged in"; header('location: index.php'); // echo "login success"; }else { array_push($errors, "Wrong username/password"); } } } ?>
Step 5:- Create Home Page
<?php session_start(); if (!isset($_SESSION['username'])) { $_SESSION['msg'] = "You must log in first"; header('location: login.php'); } if (isset($_GET['logout'])) { session_destroy(); unset($_SESSION['username']); header("location: login.php"); } ?> <!DOCTYPE html> <html> <head> <title>Home</title><!-- -----------title----- --> <!-- -------------custum css link---------------- --> <link rel="stylesheet" type="text/css" href="style.css"> </head><!-- --------------head --> <body><!-- --------------body --> <div class="header"> <h2>Home Page</h2> </div> <div class="content"> <?php if (isset($_SESSION['success'])) : ?> <div class="error success" > <h3> <?php echo $_SESSION['success']; unset($_SESSION['success']); ?> </h3> </div> <?php endif ?> <?php if (isset($_SESSION['username'])) : ?> <p>Welcome <strong><?php echo $_SESSION['username']; ?></strong></p> <p> <a href="index.php?logout='1'" style="color: red;">logout</a> </p> <?php endif ?> </div> </body> </html>
Step 6:- style file
* { margin: 0px; padding: 0px; } body { font-size: 120%; background: #F8F8FF; font-family: cursive; } .header { width: 30%; margin: 50px auto 0px; color: white; background: #1c94fb !important; text-align: center; border: 1px solid #B0C4DE; border-bottom: none; border-radius: 10px 10px 0px 0px; padding: 20px; } form, .content { width: 30%; margin: 0px auto; padding: 20px; border: 1px solid #B0C4DE; background: white; border-radius: 0px 0px 10px 10px; } .input-group { margin: 10px 0px 10px 0px; } .input-group label { display: block; text-align: left; margin: 3px; } .input-group input { height: 30px; width: 93%; padding: 5px 10px; font-size: 16px; border-radius: 5px; border: 1px solid gray; } .btn { padding: 10px; font-size: 15px; color: white; background: #1c94fb !important; border: none; border-radius: 5px; } .error { width: 92%; margin: 0px auto; padding: 10px; border: 1px solid #ff0500; color: #ff0500; background: #fff; border-radius: 5px; text-align: left; } .success { color: #3c763d; background: #fff; border: 1px solid #3c763d; margin-bottom: 20px; }