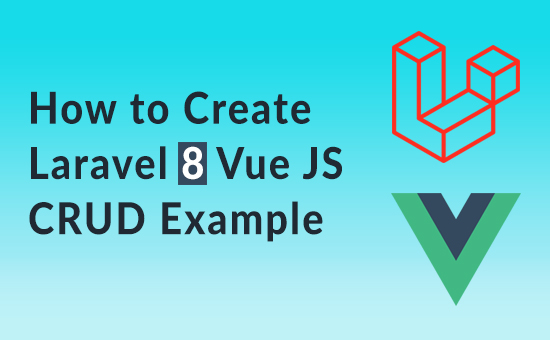
This post was last updated on May 31st, 2021 at 04:54 am
How to Create Laravel 8 Vue JS CRUD, I will show you how to implement vue js crud example with Laravel 8.
how to Create a crude API in Laravel 8, for example Vue.Js Spa Crude in Laravel 8.
In this laravel 8 vue js crud example, you will learn how to implement the laravel 8 vue js crud (create, read, update and delete) spa with Vue js router and laravel 8.
Laravel 8 Vue JS CRUD (SPA) Example
- Download Laravel 8
- Configure Database
- Create Migration, Model and Controller
- Install Laravel Vue UI
- Add Routes In web.php
- Add Vue Js Components
- Add Vue Js Routes
- Add Vue App.js
- Run Server
Download And Install Laravel
composer create-project --prefer-dist laravel/laravel blog
Step 2:- Configure Database
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel DB_USERNAME=root DB_PASSWORD=laravel@123
Create Migration, Model and Controller
Create Model
php artisan make:model Post -m
Add code in database/migrations/create_posts_table.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreatePostsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('posts', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('title'); $table->text('description'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('posts'); } }
Add Post table values in app/Models/Post.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Post extends Model { use HasFactory; protected $fillable = [ 'title', 'description' ]; }
Migrate the database using the command
php artisan migrate
Create Post Controller
php artisan make:controller PostController
open PostController and define index, add, edit, delete methods in PostController.php, go to app\Http\Controllers\PostController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Post; //codeplaners class PostController extends Controller { // all posts public function index() { $posts = Post::all()->toArray(); return array_reverse($posts); } // add post public function add(Request $request) { $post = new Post([ 'title' => $request->input('title'), 'description' => $request->input('description') ]); $post->save(); return response()->json('post successfully added'); } // edit post public function edit($id) { $post = Post::find($id); return response()->json($post); } // update post public function update($id, Request $request) { $post = Post::find($id); $post->update($request->all()); return response()->json('post successfully updated'); } // delete post public function delete($id) { $post = Post::find($id); $post->delete(); return response()->json('post successfully deleted'); } }
Add Routes in Web.php
Routes/web.php
<?php Route::get('{any}', function () { return view('app'); })->where('any', '.*');
Routes/Api.php
<?php use Illuminate\Http\Request; use Illuminate\Support\Facades\Route; use App\Http\Controllers\PostController; Route::get('posts', [PostController::class, 'index']); Route::group(['prefix' => 'post'], function () { Route::post('add', [PostController::class, 'add']); Route::get('edit/{id}', [PostController::class, 'edit']); Route::post('update/{id}', [PostController::class, 'update']); Route::delete('delete/{id}', [PostController::class, 'delete']); });
Install Laravel Vue UI
Run the following command and install Vue UI in laravel
composer require laravel/ui
php artisan ui vue
next step, use the command and install the vue-router and Vue-Axios will be used for calling Laravel API.
npm install vue-router vue-axios --save
and install npm
npm install
Now after this we run this command
npm run watch
npm run watch with this command compiles the assets.
Step 6:- Add Vue Js
To setup Vue js in Laravel, create one blade view file named app.blade.php
update the code into your app.blade.php file as follow.
resources/views/layout/app.blade.php
<!doctype html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <meta name="csrf-token" value="{{ csrf_token() }}"/> <title>Build Laravel Vue JS CRUD Example - CodePalners</title> <link href="{{ mix('css/app.css') }}" type="text/css" rel="stylesheet"/> <style> .bg-light { background-color: #eae9e9 !important; } </style> </head> <body> <div id="app"></div> <script src="{{ mix('js/app.js') }}" type="text/javascript"></script> </body> </html>
Step 7:- Add Vue Js Components
Create Components folder this path resources/js/components
and components folder add file:
- App.vue
- AllPost.vue
- AddPost.vue
- EditPost.vue
Open App.vue file and update the code your file:-
<template> <div class="container"> <div class="text-center" style="margin: 20px 0px 20px 0px;"> <span class="text-secondary">Build Laravel Vue JS CRUD Example - CodePalners</span> </div> <nav class="navbar navbar-expand-lg navbar-light bg-light"> <div class="collapse navbar-collapse"> <div class="navbar-nav"> <router-link to="/" class="nav-item nav-link">Home</router-link> <router-link to="/add" class="nav-item nav-link">Add Post</router-link> </div> </div> </nav> <br/> <router-view></router-view> </div> </template> <script> export default {} </script>
Open AllPost.vue file and update the code your file:-
<template> <div> <h3 class="text-center">All Posts</h3><br/> <table class="table table-bordered"> <thead> <tr> <th>ID</th> <th>Title</th> <th>Description</th> <th>Created At</th> <th>Updated At</th> <th>Actions</th> </tr> </thead> <tbody> <tr v-for="post in posts" :key="post.id"> <td>{{ post.id }}</td> <td>{{ post.title }}</td> <td>{{ post.description }}</td> <td>{{ post.created_at }}</td> <td>{{ post.updated_at }}</td> <td> <div class="btn-group" role="group"> <router-link :to="{name: 'edit', params: { id: post.id }}" class="btn btn-primary">Edit </router-link> <button class="btn btn-danger" @click="deletePost(post.id)">Delete</button> </div> </td> </tr> </tbody> </table> </div> </template> <script> export default { data() { return { posts: [] } }, created() { this.axios .get('http://127.0.0.1:8000/api/posts') .then(response => { this.posts = response.data; }); }, methods: { deletePost(id) { this.axios .delete(`http://127.0.0.1:8000/api/post/delete/${id}`) .then(response => { let i = this.posts.map(item => item.id).indexOf(id); // find index of your object this.posts.splice(i, 1) }); } } } </script>
Open AddPost.vue file and update the code your file:-
<template> <div> <h3 class="text-center">Add Post</h3> <div class="row"> <div class="col-md-6"> <form @submit.prevent="addPost"> <div class="form-group"> <label>Title</label> <input type="text" class="form-control" v-model="post.title"> </div> <div class="form-group"> <label>Description</label> <input type="text" class="form-control" v-model="post.description"> </div> <button type="submit" class="btn btn-primary">Add Post</button> </form> </div> </div> </div> </template> <script> export default { data() { return { post: {} } }, methods: { addPost() { this.axios .post('http://127.0.0.1:8000/api/post/add', this.post) .then(response => ( this.$router.push({name: 'home'}) // console.log(response.data) )) .catch(error => console.log(error)) .finally(() => this.loading = false) } } } </script>
Open EditPost.vue file and update the code your file:-
<template> <div> <h3 class="text-center">Edit Post</h3> <div class="row"> <div class="col-md-6"> <form @submit.prevent="updatePost"> <div class="form-group"> <label>Title</label> <input type="text" class="form-control" v-model="post.title"> </div> <div class="form-group"> <label>Description</label> <input type="text" class="form-control" v-model="post.description"> </div> <button type="submit" class="btn btn-primary">Update Post</button> </form> </div> </div> </div> </template> <script> export default { data() { return { post: {} } }, created() { this.axios .get(`http://127.0.0.1:8000/api/post/edit/${this.$route.params.id}`) .then((response) => { this.post = response.data; // console.log(response.data); }); }, methods: { updatePost() { this.axios .post(`http://127.0.0.1:8000/api/post/update/${this.$route.params.id}`, this.post) .then((response) => { this.$router.push({name: 'home'}); }); } } } </script>
Step 8:- Add Vue Js Routes
In this folder resources>js add routes.js file.
Open routes.js file and update the code your file:-
import AllPosts from './components/AllPost.vue'; import AddPost from './components/AddPost.vue'; import EditPost from './components/EditPost.vue'; export const routes = [ { name: 'home', path: '/', component: AllPosts }, { name: 'add', path: '/add', component: AddPost }, { name: 'edit', path: '/edit/:id', component: EditPost } ];
Step 9:- Add Vue App.js
this final step, please add the following code in the resources/js/app.js file:-
require('./bootstrap'); import Vue from 'vue'; window.Vue = require('vue'); import App from './components/App.vue'; import VueRouter from 'vue-router'; import VueAxios from 'vue-axios'; import axios from 'axios'; import {routes} from './routes'; Vue.use(VueRouter); Vue.use(VueAxios, axios); const router = new VueRouter({ mode: 'history', routes: routes }); const app = new Vue({ el: '#app', router: router, render: h => h(App), });
Step 9:- Run Laravel Vue CRUD App
npm run watch
php artisan serve
Open the URL in the browser:
http://127.0.0.1:8000