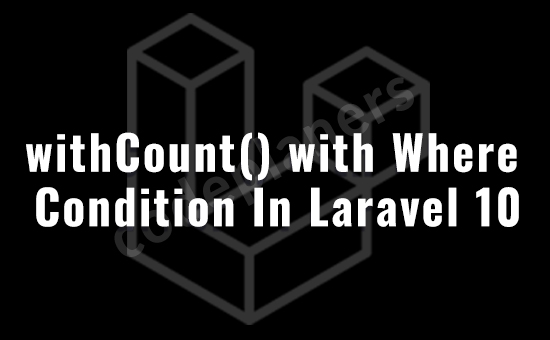
Hi dev,
Today, i show you withCount() with where condition in laravel 10. This article will give you simple example of withCount() with where condition in laravel 10. you will withCount() with where condition in laravel 10. In this article, we will implement a withCount() with where condition in laravel 10.
you can easily use withCount() with laravel 6, laravel 7, laravel 8, laravel 9 and laravel 10 version.
So, let’s follow few steps to create example of withCount() with where condition in laravel 10.
Category Model:
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Category extends Model { use HasFactory; /** * Get the comments for the blog post. */ public function products() { return $this->hasMany(Product::class); } }
Product Model:
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; protected $fillable = [ 'name', 'price', 'is_active' ]; }
withCount() Example:
<?php namespace App\Http\Controllers; use App\Models\Category; class SignaturePadController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $categories = Category::select("id", "name") ->withCount('products') ->get() ->toArray(); dd($categories); } }
Output:
Array ( [0] => Array ( [id] => 1 [name] => Mobile [products_count] => 3 ) [1] => Array ( [id] => 2 [name] => Laptop [products_count] => 2 ) )
withCount() with Where Condition Example:
<?php namespace App\Http\Controllers; use App\Models\Category; class SignaturePadController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $categories = Category::select("id", "name") ->withCount([ 'products as active_products_count' => function ($query) { $query->where('is_active', '1'); }, ]) ->get() ->toArray(); dd($categories); } }
Output:
Array ( [0] => Array ( [id] => 1 [name] => Mobile [active_products_count] => 2 ) [1] => Array ( [id] => 2 [name] => Laptop [active_products_count] => 2 ) )
I hope it will assist you…