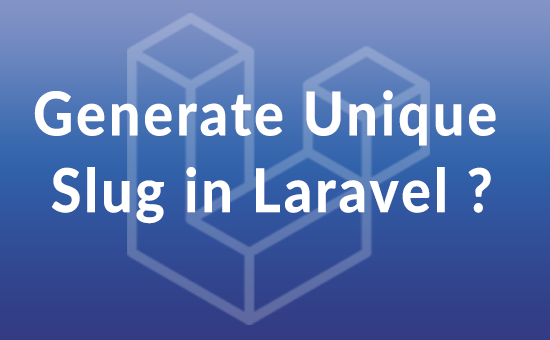
In this article, we will show you Generate Unique Slug in Laravel. This article will give you simple example of Generate Unique Slug in Laravel. you will learn Generate Unique Slug in Laravel.
This article will give you simple example of Generate Unique Slug in Laravel of how to add Unique Slug in laravel 6, laravel 7 and laravel 8 version.
app/Models/Product.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; use Illuminate\Support\Str; class Product extends Model { use HasFactory; protected $fillable = [ 'title', 'detail', 'slug' ]; /** * Boot the model. */ protected static function boot() { parent::boot(); static::created(function ($product) { $product->slug = $product->createSlug($product->title); $product->save(); }); } /** * Write code on Method * * @return response() */ private function createSlug($title){ if (static::whereSlug($slug = Str::slug($title))->exists()) { $max = static::whereTitle($title)->latest('id')->skip(1)->value('slug'); if (is_numeric($max[-1])) { return preg_replace_callback('/(\d+)$/', function ($mathces) { return $mathces[1] + 1; }, $max); } return "{$slug}-2"; } return $slug; } }
Controller Code
<?php namespace App\Http\Controllers; use App\Models\Product; use Illuminate\Http\Request; class ProductController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $products = Product::create([ "title" => "Laravel 8 post Upload" ]); dd($products); } }
now if you create multiple time same title record then it will create slug as bellow.
laravel-8-post-upload laravel-8-post-upload-2 laravel-8-post-upload-3 laravel-8-post-upload-4