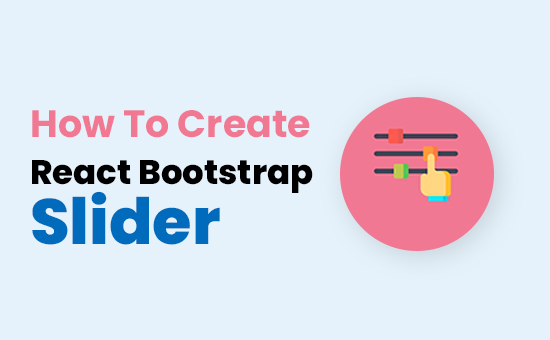
Interoduction
The Build React App was used to bootstrap this project. It was then ejected because of a problem with jQuery (see below).
A ReactJS wrapper with Bootstrap for the Seiyria Bootstrap Slider part. Start with version 3.0.0, we only support Bootstrap 4. If you are still using Bootstrap 3, please use version 2.2.3.
How to install
Install from npm with:
npm install --save react-bootstrap-slider
or
yarn add react-bootstrap-slider
Import like so for ES6:
import ReactBootstrapSlider from 'react-bootstrap-slider';
The control is implemented in the UMD format, so AMD / RequireJS should also function, but I haven’t checked it.
Peer Dependencies
For react-bootstrap-slider, React, React Dom, and Bootstrap are classified as peer dependencies (peerDependencies section of package.json). When you install this component in your own project, it won’t be automatically installed. If you have not already done so, you will need to install them yourself, as part of that project. If you do not include them yet, this command will install them for you:
npm install react react-dom prop-types bootstrap@^4 --save
or
yarn add react react-dom prop-types bootstrap@^4
jQuery
If it detects it in your project configuration, the bootstrap-slider part-and thus, react-bootstrap-slider-will work with jQuery, but it isn’t a necessity. Without jQuery, it worked great. However, if you are using Webpack or Browserify to create your project, if jQuery is not present, you can get a “missing dependency” create error. This is a known upstream bootstrap-slider problem. See How do I remove the JQuery optional dependency from my build? Readme f on the Bootstrap Slider’s on the Bootstrap Slider on how this problem can be worked around. By reviewing the /examples / config / webpack.config.js file and searching for ‘jQuery, you can also see how I did it.
You also need to make sure you have included the CSS file of the bootstrap-slider in your build, otherwise the control will be null! Of course, you’ll also need Bootstrap’s own CSS file as well. If you are using Webpack, which you would be if you have a project based on Create React App, then you can directly import the CSS file into your build, like this:
import "bootstrap/dist/css/bootstrap.css";
import "bootstrap-slider/dist/css/bootstrap-slider.css"
Those two imports need to be in your top-level JavaScript file, e.g. App.js.
Or you can simply add the files as links in your HTML file as a link e.g.:
<link rel="stylesheet" href="//stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" />
<script src="//cdnjs.cloudflare.com/ajax/libs/bootstrap-slider/11.0.2/bootstrap-slider.min.js"></script>
Checking that the versions match those of Bootstrap and Bootstrap Slider themselves.
How to create react-bootstrap-slider
Here’s an example of how you might call it in your ReactJS’s render method:
<ReactBootstrapSlider
value={this.state.currentValue}
change={this.changeValue}
slideStop={this.changeValue}
step={this.state.step}
max={this.state.max}
min={this.state.min}
orientation="vertical"
reversed={true}
disabled="disabled" />
The value, step, max, and min parameters should be self-explanatory. The change
event is the callback method that will be called when the slider actually changes. (NB: this is the change
event for the Bootstrap slider.) As of version 1.1.2, there is a new slideStop
event, which only triggers when you’ve finished moving the slider (i.e. you release it). This makes slideStop
a deal less “chatty” than the change
event. Decide which one you need; you’ll only ever need one or the other (unlike my example code above!).
If the optional parameter disabled is included, and is set to “disabled”, then the slider will display in a disabled state. If the parameter is not included, or is set to anything else except “disabled”, then the slider control will be enabled.
The default orientation value is ‘horizontal’, so you only need to include that prop if you want to set it to ‘vertical’.
If value is a two member array, then the slider will have two handles: minimum and maximum. You should also pass win a range parameter set to true in this case.
As of version 1.0.5, other values passed into the component will also passed into the underlying bootstrap-slider component via the Object Spread Operator that’s been proposed for ES2017 (yes, that’s how cutting edge I am!!). See seyria’s documentation for a full list of these. Those parameters have not all been tested yet but the reversed
parameter definitely works, and I have included this in the demo of the vertical slider.
Version 1.0.6 fixed an issue that prevented ticks props from being rendered. Pass in ticks props like so:
ticks = {[0, 100, 200, 300, 400]}
ticks_labels = {["$0", "$100", "$200", "$300", "$400"]}
ticks_snap_bounds = { 30 }
Current Tags
- 3.0.0 … latest (4 months ago)
28 Versions
- 3.0.0 … 4 months ago
- 2.2.3 … 9 months ago
- 2.2.2 … 2 years ago
- 2.2.1 … 2 years ago
- 2.2.0 … 2 years ago
- 2.1.5 … 2 years ago
- 2.1.4 … 3 years ago
- 2.1.3 … 3 years ago
- 2.1.2 … 3 years ago
- 2.1.1 … 3 years ago
- 2.1.0 … 3 years ago
- 2.0.1 … 3 years ago
- 2.0.0 … 3 years ago
- 1.1.7 … 4 years ago
- 1.1.6 … 4 years ago
- 1.1.5 … 4 years ago
- 1.1.4 … 4 years ago
- 1.1.3 … 4 years ago
- 1.1.2 … 4 years ago
- 1.1.1 … 4 years ago
- 1.1.0 … 4 years ago
- 1.0.6 … 4 years ago
- 1.0.5 … 4 years ago
- 1.0.4 … 4 years ago
- 1.0.3 … 4 years ago
- 1.0.2 … 4 years ago
- 1.0.1 … 4 years ago
- 1.0.0 … 5 years ago