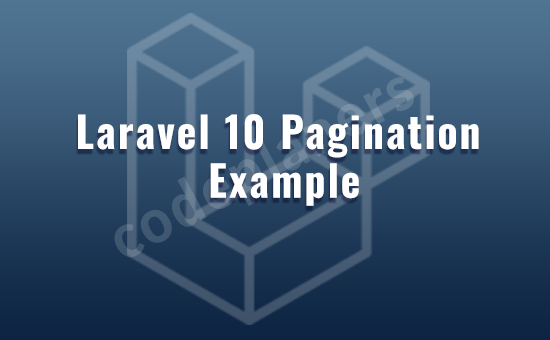
Hi Dev,
Are you trying to find an example of pagination with Laravel 10? It is a straightforward example of laravel 10 pagination using bootstrap 5. The idea behind the laravel 10 pagination tailwind example is clear. We’ll examine a case study demonstrating how to install pagination in Laravel 10. So, let’s examine an example in more detail.
We’ll conduct the migration in this example and make a “users” table. The tinker command will then be used to produce fake records. Then we will utilise pagination to display those users. By default, Laravel uses the Tailwind CSS design for pagination; in this article, we’ll utilise the Bootstrap 5 design.
So let’s follow few step.
Step 1: Install Laravel 10
Run command and install laravel
composer create-project laravel/laravel example-app
Step 2: Database Configuration
.env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=here your database name DB_USERNAME=here database username DB_PASSWORD=here database password
Step 3: Create Dummy Users
Let’s run migration command:
php artisan migrate
Next, run ticker command to add dummy users:
php artisan tinker User::factory()->count(100)->create()
Step 4: Add Route
routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\UserController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('users', [UserController::class, 'index']);
Step 5: Create Controller
app/Http/Controllers/UserController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Illuminate\View\View; class UserController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(Request $request): View { $users = User::paginate(5); return view('users', compact('users')); } }
Step 6: Create Blade File
resources/views/users.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel 10 Pagination Example - ItSolutionStuff.com</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.0.1/css/bootstrap.min.css" rel="stylesheet"> </head> <body> <div class="container"> <h1>Laravel 10 Pagination Example - ItSolutionStuff.com</h1> <table class="table table-bordered data-table"> <thead> <tr> <th>ID</th> <th>Name</th> <th>Email</th> </tr> </thead> <tbody> @forelse($users as $user) <tr> <td>{{ $user->id }}</td> <td>{{ $user->name }}</td> <td>{{ $user->email }}</td> </tr> @empty <tr> <td colspan="3">There are no users.</td> </tr> @endforelse </tbody> </table> <!-- You can use Tailwind CSS Pagination as like here: {!! $users->withQueryString()->links() !!} --> {!! $users->withQueryString()->links('pagination::bootstrap-5') !!} </div> </body> </html>
Run Laravel App:
All the required steps have been done, now you have to type the given below command and hit enter to run the Laravel app:
php artisan serve
http://localhost:8000/users
I hope it will assist you…