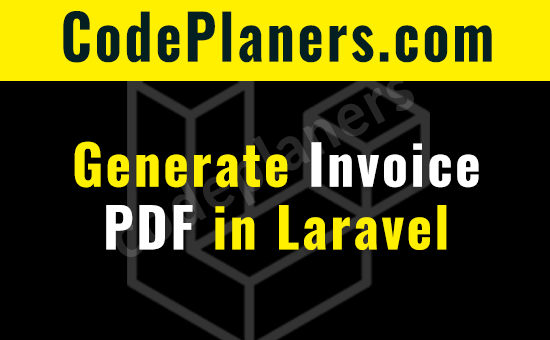
Hi Dev,
Today, we will show you Generate Invoice PDF in Laravel. This article will give you simple example of Generate Invoice PDF in Laravel. Let’s discuss Generate Invoice PDF in Laravel. In this article, we will implement a Generate Invoice PDF in Laravel.
use this example with laravel 6, laravel 7, laravel 8, laravel 9 and laravel 10 versions.
So let’s follow few step to create example of Generate Invoice PDF in Laravel.
- Step 1:Install Laravel
- Step 2: Install DomPDF Package
- Step 3: Create Controller
- Step 4: Add Route
- Step 5: Create View File
- Run Laravel
Step 1: Install Laravel
composer create-project laravel/laravel example-app
Step 2: Install DomPDF Package
composer require barryvdh/laravel-dompdf
Step 3: Create Controller
php artisan make:controller InvoiceController
app/Http/Controllers/InvoiceController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Barryvdh\DomPDF\Facade\Pdf; class InvoiceController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $data = [ [ 'quantity' => 2, 'description' => 'Gold', 'price' => '$500.00' ], [ 'quantity' => 3, 'description' => 'Silver', 'price' => '$300.00' ], [ 'quantity' => 5, 'description' => 'Platinum', 'price' => '$200.00' ] ]; $pdf = Pdf::loadView('invoice', ['data' => $data]); return $pdf->download(); } }
Step 4: Add Route
routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\InvoiceController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('invoice-pdf', [InvoiceController::class, 'index']);
Step 5: Create View File
resources/views/invoice.blade.php
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Code Planers</title> <link rel="stylesheet" href="{{ public_path('invoice.css') }}" type="text/css"> </head> <body> <table class="table-no-border"> <tr> <td class="width-70"> <img src="{{ public_path('codeplaners.png') }}" alt="" width="200" /> </td> <td class="width-30"> <h2>Invoice ID: 7888573</h2> </td> </tr> </table> <div class="margin-top"> <table class="table-no-border"> <tr> <td class="width-50"> <div><strong>To:</strong></div> <div>Code Planers</div> <div>1568 NW 4th Path, RAJ - 302013</div> <div><strong>Phone:</strong> (325) 781-1861</div> <div><strong>Email:</strong> info@gmail.com</div> </td> <td class="width-50"> <div><strong>From:</strong></div> <div>Mohan Yadav</div> <div>174, Jaipur, Rajasthan - 302039</div> <div><strong>Phone:</strong> 9829601023</div> <div><strong>Email:</strong> codeplaners@gmail.com</div> </td> </tr> </table> </div> <div> <table class="product-table"> <thead> <tr> <th class="width-25"> <strong>Qty</strong> </th> <th class="width-50"> <strong>Product</strong> </th> <th class="width-25"> <strong>Price</strong> </th> </tr> </thead> <tbody> @foreach($data as $value) <tr> <td class="width-25"> {{ $value['quantity'] }} </td> <td class="width-50"> {{ $value['description'] }} </td> <td class="width-25"> {{ $value['price'] }} </td> </tr> @endforeach </tbody> <tfoot> <tr> <td class="width-70" colspan="2"> <strong>Sub Total:</strong> </td> <td class="width-25"> <strong>$1000.00</strong> </td> </tr> <tr> <td class="width-70" colspan="2"> <strong>Tax</strong>(10%): </td> <td class="width-25"> <strong>$100.00</strong> </td> </tr> <tr> <td class="width-70" colspan="2"> <strong>Total Amount:</strong> </td> <td class="width-25"> <strong>$1100.00</strong> </td> </tr> </tfoot> </table> </div> <div class="footer-div"> <p>Thank you, <br/>@CodePlaners.com</p> </div> </body> </html>
public/invoice.css
body{ font-family: system-ui, system-ui, sans-serif; } table{ border-spacing: 0; } table td, table th, p{ font-size: 13px !important; } img{ border: 3px solid #F1F5F9; padding: 6px; background-color: #F1F5F9; } .table-no-border{ width: 100%; } .table-no-border .width-50{ width: 50%; } .table-no-border .width-70{ width: 70%; text-align: left; } .table-no-border .width-30{ width: 30%; } .margin-top{ margin-top: 40px; } .product-table{ margin-top: 20px; width: 100%; border-width: 0px; } .product-table thead th{ background-color: #60A5FA; color: white; padding: 5px; text-align: left; padding: 5px 15px; } .width-20{ width: 20%; } .width-50{ width: 50%; } .width-25{ width: 25%; } .width-70{ width: 70%; text-align: right; } .product-table tbody td{ background-color: #F1F5F9; color: black; padding: 5px 15px; } .product-table td:last-child, .product-table th:last-child{ text-align: right; } .product-table tfoot td{ color: black; padding: 5px 15px; } .footer-div{ background-color: #F1F5F9; margin-top: 100px; padding: 3px 10px; }
Run Laravel App
php artisan serve
I hope it will assist you…