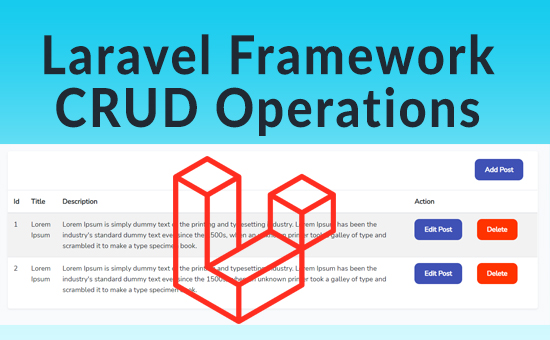
Laravel Framework CRUD Operations, I will show you how to implement Operations crud example with Laravel.
In this laravel crud example, you will learn how to implement the laravel crud (create, read, update and delete) .
Laravel CRUD Operations
- Download Laravel
- Configure Database
- Create Migration, Model and Controller
- Add Routes In web.php
- Run Server
Step 1:- Download And Install Laravel
composer create-project --prefer-dist laravel/laravel blog
Step 2:- Configure Database
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel DB_USERNAME=root DB_PASSWORD=
Step 3:- Create Migration, Model and Controller
Create Model
php artisan make:model Post -m
Add code in database/migrations/create_posts_table.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreatePostsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('posts', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('title'); $table->text('description'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('posts'); } }
Add Post table values in app/Models/Post.php
<?php namespace App; use Illuminate\Database\Eloquent\Model; class Post extends Model { protected $fillable = ['title', 'description']; }
Migrate the database using the command
php artisan migrate
Create Post Controller
php artisan make:controller PostController
open PostController and define index, add, edit, delete methods in PostController.php, go to app\Http\Controllers\PostController.php
<?php namespace App\Http\Controllers; use DB; use Session; use App\Models\Post; use Illuminate\Http\Request; class PostController extends Controller { // all posts public function index() { $posts = Post::all(); return view('post/index',compact('posts',$posts)); } // post add public function AddPost(Request $request) { return view('post/add-post'); } // post Store public function Store(Request $request){ $this->validate($request, [ 'title' => 'required|string|min:3|max:255', 'description' => 'required', ]); $pages = new Post; $pages->title = $request['title']; $pages->description = $request['description']; $pages->save(); Session::flash( 'message', 'Post published.' ); return redirect('/post'); } // post posteditshow public function PostShow($id) { $posts = DB::select('select * from posts where id = ?',[$id]); return view('post/postedit',['posts'=>$posts]); } // post Edit public function Postedit(Request $request,$id) { $request->validate([ 'title' => 'required', ]); $update['title'] = $request->get('title'); $update['description'] = $request->get('description'); Post::where('id',$id)->update($update); $request->session()->flash('message', 'Successfully Updated!'); return redirect('/post'); } // post Delete public function Delete(Request $request,$id) { DB::delete('delete from posts where id = ?',[$id]); $request->session()->flash('message', 'Successfully Delete!'); return redirect('/post'); } }
Step 4:- Add post folder in resources
Create post folder this path resources\views
and post folder add file:
- index.blade.php
- add-post.blade.php
- postedit.blade.php
Open index.blade.php file and update the code your file:-
@extends('layouts.app') @section('content') <div class="bg-white table-responsive rounded shadow-sm pt-3 pb-3 mb-30"> <div><a href="{{ url('add-post') }}" class="btn">Add Post</a></div> @if(Session::has('message')) <p class="alert alert-info" style="clear: both;">{{ Session::get('message') }}</p> @endif <table id="data-table" class="table mb-0 table-striped" cellspacing="0" width="100%"> <thead> <tr> <th data-orderable="false">Id</th> <th>Title</th> <th>Description</th> <th width="270">Action</th> </tr> </thead> <tbody> @foreach ($posts as $post) <tr> <td>{{ $post->id }}</td> <td>{{ $post->title }}</td> <td>{{ $post->description }}</td> <td><a href="{{ url('postedit') }}/{{ $post->id }}" class="btn" style="float: left;">Edit Post</a><a href="postdelete/{{ $post->id }}" class="btn" style="background:#F30;">Delete</a></td> </tr> @endforeach </tbody> </table> </div> @endsection
Open add-post.blade.php file and update the code your file:-
@extends('layouts.app') @section('content') <div class="page-content"> <div class="container-fluid"> <div class="row"> <div class="col-lg-12"> <div class="portlet-box portlet-gutter ui-buttons-col mb-30"> <div class="portlet-body"> <form class="needs-validation" method="post" action="{{ url('add-post') }}" novalidate enctype="multipart/form-data" > <input type = "hidden" name = "_token" value = "<?php echo csrf_token(); ?>"> <div class="form-row"> <div class="col-md-12 mb-3"> <label for="validationCustom01">Post Title</label> <input type="text" class="form-control" name="title" placeholder="Page Title" > </div> <div class="col-md-12 mb-3"> <label for="image">Post Description</label> <textarea id="description" class="form-control" name="description" ></textarea> </div> </div> <button class="btn btn-primary" type="submit">Submit</button> </form> </div> </div> </div> </div> </div> </div> @endsection
Open postedit.blade.php file and update the code your file:-
@extends('layouts.app') @section('content') <div class="page-content"> <div class="container-fluid"> <div class="row"> <div class="col-lg-12"> <div class="portlet-box portlet-gutter ui-buttons-col mb-30"> <div class="portlet-body"> <form class="needs-validation" method="post" action="{{ url('postedit') }}/<?php echo $posts[0]->id; ?>" novalidate enctype="multipart/form-data" > <input type = "hidden" name = "_token" value = "<?php echo csrf_token(); ?>"> <div class="form-row"> <div class="col-md-12 mb-3"> <label for="validationCustom01">Post Title</label> <input type="text" class="form-control" name="title" placeholder="Page Title" value = '<?php echo $posts[0]->title; ?>' > </div> <div class="col-md-12 mb-3"> <label for="image">Post Description</label> <textarea id="description" class="form-control" name="description" ><?php echo $posts[0]->description; ?></textarea> </div> </div> <button class="btn btn-primary" type="submit">Submit</button> </form> </div> </div> </div> </div> </div> </div> @endsection
Step 5:- Add Routes in Web.php
Routes/web.php
Route::get('/post', 'PostController@index'); Route::get('/add-post', 'PostController@AddPost'); Route::post('/add-post','PostController@Store'); Route::get('/postedit/{id}', 'PostController@PostShow'); Route::post('/postedit/{slug}', 'PostController@Postedit'); Route::get('/postdelete/{id}', 'PostController@Delete');
Step 6:- Run Laravel Vue CRUD App
php artisan serve
Open the URL in the browser:
http://127.0.0.1:8000