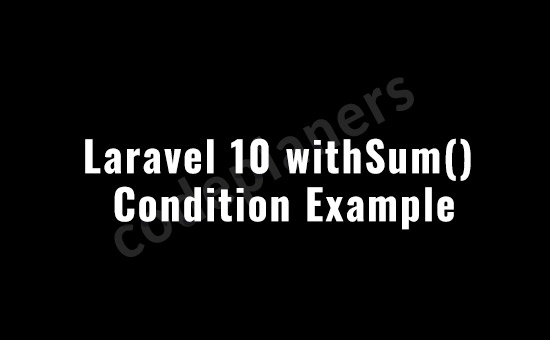
Hi dev,
Today, i show you laravel 10 withSum() condition example. This article will give you simple example of laravel 10 withSum() condition example. you will laravel 10 withSum() condition example. In this article, we will implement a laravel 10 withSum() condition example.
you can easily use withSum() with laravel 6, laravel 7, laravel 8, laravel 9 and laravel 10 version.
So, let’s follow few steps to create example of laravel 10 withSum() condition example.
Category Model:
<?php <?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Category extends Model { use HasFactory; /** * Get the comments for the blog post. */ public function products() { return $this->hasMany(Product::class); } }
Product Model:
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; protected $fillable = [ 'name', 'price', 'is_active' ]; }
withSum() Example:
<?php namespace App\Http\Controllers; use App\Models\Category; class SignaturePadController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $categories = Category::select("id", "name") ->withSum('products', 'price') ->get() ->toArray(); dd($categories); } }
Output:
Array ( [0] => Array ( [id] => 1 [name] => Mobile [products_sum_price] => 30 ) [1] => Array ( [id] => 2 [name] => Laptop [products_sum_price] => 20 ) )
withSum() with Where Condition Example:
<?php namespace App\Http\Controllers; use App\Models\Category; class SignaturePadController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $categories = Category::select("id", "name") ->withSum([ 'products' => function ($query) { $query->where('is_active', '1'); }], 'price') ->get() ->toArray(); dd($categories); } }
Output:
Array ( [0] => Array ( [id] => 1 [name] => Mobile [products_sum_price] => 20 ) [1] => Array ( [id] => 2 [name] => Laptop [products_sum_price] => 20 ) )
I hope it will assist you…