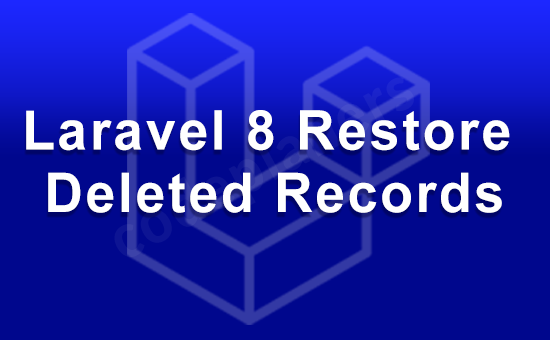
Hi Dev,
Today, i we will show you laravel 8 restore deleted records. This article will give you simple example of laravel 8 restore deleted records. you will learn laravel 8 restore deleted records. So let’s follow few step to create example of laravel 8 restore deleted records.
Step 1: Install Laravel
composer create-project --prefer-dist laravel/laravel blog
Step 2: Add SoftDelete in User Model
php artisan make:migration add_sorft_delete_column
database/migrations/add_sorft_delete_column.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class AddSorftDeleteColumn extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('users', function(Blueprint $table){ $table->softDeletes(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::table('users', function (Blueprint $table) { $table->dropSoftDeletes(); }); } }
run migration:
php artisan migrate
app/Models/User.php
<?php namespace App\Models; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Illuminate\Database\Eloquent\SoftDeletes; class User extends Authenticatable { use HasFactory, Notifiable, SoftDeletes; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password' ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast to native types. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; }
Step 3: Add Dummy Users
php artisan tinker \App\Models\User::factory(10)->create();
Step 4: Create Route
need to create some routes for add to cart function.
routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\UserController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('users', [UserController::class, 'index'])->name('users.index'); Route::delete('users/{id}', [UserController::class, 'delete'])->name('users.delete'); Route::get('users/restore/one/{id}', [UserController::class, 'restore'])->name('users.restore');
Step 5: Create Controller
app/Http/Controllers/UserController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(Request $request) { $users = User::select("*"); if ($request->has('view_deleted')) { $users = $users->onlyTrashed(); } $users = $users->paginate(8); return view('users', compact('users')); } /** * Write code on Method * * @return response() */ public function delete($id) { User::find($id)->delete(); return back(); } /** * Write code on Method * * @return response() */ public function restore($id) { User::withTrashed()->find($id)->restore(); return back(); } /** * Write code on Method * * @return response() */ public function restoreAll() { User::onlyTrashed()->restore(); return back(); } }
Step 6: Create Blade Files
need to create blade files for users
resources/views/users.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel 8 Restore Deleted Records</title> <meta name="csrf-token" content="{{ csrf_token() }}"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script> </head> <body> <div class="container"> <h1>Laravel 8 Restore Deleted Records</h1> @if(request()->has('view_deleted')) <a href="{{ route('users.index') }}" class="btn btn-info">View All Users</a> <a href="{{ route('users.restore.all') }}" class="btn btn-success">Restore All</a> @else <a href="{{ route('users.index', ['view_deleted' => 'DeletedRecords']) }}" class="btn btn-primary">View Delete Records</a> @endif <table class="table table-bordered data-table"> <thead> <tr> <th>No</th> <th>Name</th> <th>Email</th> <th>Action</th> </tr> </thead> <tbody> @foreach($users as $user) <tr> <td>{{ $user->id }}</td> <td>{{ $user->name }}</td> <td>{{ $user->email }}</td> <td> @if(request()->has('view_deleted')) <a href="{{ route('users.restore', $user->id) }}" class="btn btn-success">Restore</a> @else <form method="POST" action="{{ route('users.delete', $user->id) }}"> @csrf <input name="_method" type="hidden" value="DELETE"> <button type="submit" class="btn btn-xs btn-danger btn-flat show_confirm" data-toggle="tooltip" title='Delete'>Delete</button> </form> @endif </td> </tr> @endforeach </tbody> </table> </div> </body> <script type="text/javascript"> $('.show_confirm').click(function(e) { if(!confirm('Are you sure you want to delete this?')) { e.preventDefault(); } }); </script> </html>
Now we are ready to run our example
php artisan serve