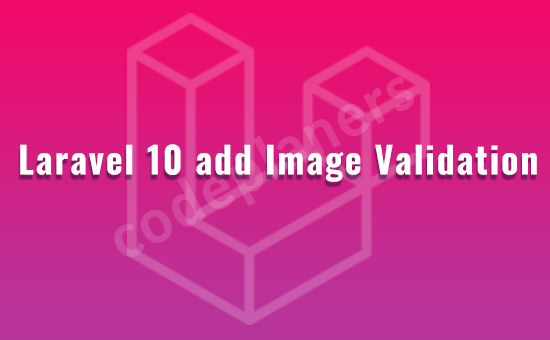
Hi dev,
Today, i show you laravel 10 add image validation. This article will give you simple example of laravel 10 add image validation. you will laravel 10 add image validation. In this article, we will implement a laravel 10 add image validation.
mimes, dimensions, and maximum
Using the aforementioned validation criteria, the file types “jpg,png,jpeg,gif,svg” and “max” may be specified, and the file size restriction can be established using max. Using dimensions, you can restrict a file’s height and width. Let’s examine the simple sample code that follows.
So, let’s follow few steps to create example of laravel 10 add image validation.
Install Laravel 10
Run command and install laravel
composer create-project laravel/laravel example-image
Create Controller
Run command and add ImageController
php artisan make:controller ImageController
app/Http/Controllers/ImageController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\View\View; use Illuminate\Http\RedirectResponse; class ImageController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(): View { return view('imageUpload'); } /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function store(Request $request): RedirectResponse { $this->validate($request, [ 'image' => ['required', 'image', 'mimes:jpg,png,jpeg,gif,svg', 'dimensions:min_width=100,min_height=100,max_width=1000,max_height=1000', 'max:2048'], ]); $imageName = time().'.'.$request->image->extension(); $request->image->move(public_path('images'), $imageName); /* Write Code Here for Store $imageName name in DATABASE from HERE */ return back() ->with('success', 'You have successfully upload image.') ->with('image', $imageName); } }
Create and Add Routes
routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\ImageController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::controller(ImageController::class)->group(function(){ Route::get('image-upload', 'index'); Route::post('image-upload', 'store')->name('image.store'); });
Create Blade File
resources/views/imageUpload.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel 10 Image Upload Example</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"> </head> <body> <div class="container"> <div class="panel panel-primary"> <div class="panel-heading"> <h2></h2> </div> <div class="panel-body"> @if ($message = Session::get('success')) <div class="alert alert-success alert-block"> <button type="button" class="close" data-dismiss="alert">×</button> <strong>{{ $message }}</strong> </div> <img src="images/{{ Session::get('image') }}"> @endif <form action="{{ route('image.store') }}" method="POST" enctype="multipart/form-data"> @csrf <div class="mb-3"> <label class="form-label" for="inputImage">Image:</label> <input type="file" name="image" id="inputImage" class="form-control @error('image') is-invalid @enderror"> @error('image') <span class="text-danger">{{ $message }}</span> @enderror </div> <div class="mb-3"> <button type="submit" class="btn btn-success">Upload</button> </div> </form> </div> </div> </div> </body> </html>
Run Laravel:
php artisan serve
http://localhost:8000/example-image
I hope it will assist you…