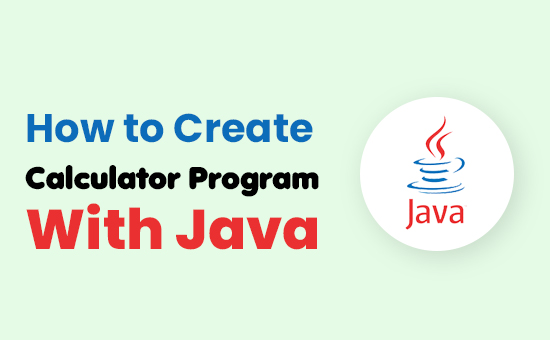
Introduction
In this code, we build a simple calculator based on the user ‘s input that performs addition , subtraction , multiplication and division. The code takes the value of both numbers (entered by the user) and then allows the user to enter the operation (+,-, * and /), which is based on the input programme that performs the selected operation using the switch case on the tried to enter numbers.
Step 1) Java Calculator with Source Code: With the help of AWT / Swing with event handling, we can create a Java calculator. Let ‘s look at the calculator development code in Java.
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
Scanner reader = new Scanner(System.in);
System.out.print("Enter two numbers: ");
// nextDouble() reads the next double from the keyboard
double first = reader.nextDouble();
double second = reader.nextDouble();
System.out.print("Enter an operator (+, -, *, /): ");
char operator = reader.next().charAt(0);
double result;
switch(operator)
{
case '+':
result = first + second;
break;
case '-':
result = first - second;
break;
case '*':
result = first * second;
break;
case '/':
result = first / second;
break;
// operator doesn't match any case constant (+, -, *, /)
default:
System.out.printf("Error! operator is not correct");
return;
}
System.out.printf("%.1f %c %.1f = %.1f", first, operator, second, result);
}
}
Step 2) Output
Enter two numbers: 2.0 3.5 Enter an operator (+, -, *, /): * 2.0 / 3.5 = 7.0
The operator * entered by the user is stored in the operator variable using the Scanner object’s next) (method.
Similarly, using the nextDouble) (method of Scanner object, the two operands, 2.0 and 3.5 are stored in variables first and second respectively.
Step 3) Since the operator / fits the condition ‘/’ when:, the programme control jumps to
result = first / second;
This statement defines the product and stores the result of the variable and the break; the declaration ends the statement of the turn.