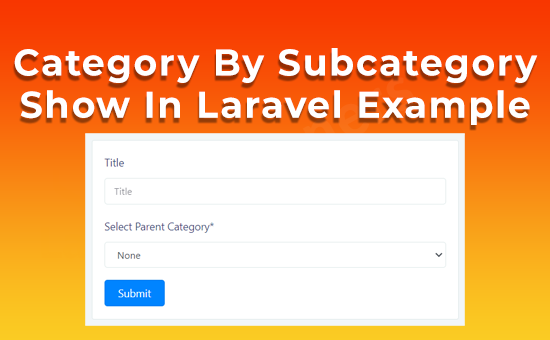
Hi Dev,
Today, i we will show you category by Subcategory show in laravel example. This article will give you simple example of category by Subcategory show in laravel example. you will category by Subcategory show in laravel example. In this article, we will implement a category by Subcategory show in laravel example.
So, let’s follow few steps to create example of category by Subcategory show in laravel example.
Preview:-
Create Model and migration
php artisan make:model Category -m
database/migrations
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateCategoriesTable extends Migration { public function up() { Schema::create('categories', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('slug')->unique(); $table->integer('parent_id')->nullable(); $table->timestamps(); }); } public function down() { Schema::dropIfExists('categories'); } }
app/Models/Category.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Category extends Model { use HasFactory; protected $fillable = ['name', 'slug', 'parent_id']; public function subcategory() { return $this->hasMany(\App\Models\Category::class, 'parent_id'); } public function parent() { return $this->belongsTo(\App\Models\Category::class, 'parent_id'); } }
Now we need to run migration.
so let’s run bellow command:
php artisan migrate
Create Routes:
Route::any('category/create', [CategoryController::class, 'createCategory'])->name('createCategory');
Create Controller:
php artisan make:controller CategoryController
<?php namespace App\Http\Controllers; use App\Models\Category; use Illuminate\Http\Request; class CategoryController extends Controller { public function createCategory(Request $request) { $categories = Category::where('parent_id', null)->orderby('name', 'asc')->get(); if($request->method()=='GET') { return view('create-category', compact('categories')); } if($request->method()=='POST') { $validator = $request->validate([ 'name' => 'required', 'slug' => 'required|unique:categories', 'parent_id' => 'nullable|numeric' ]); Category::create([ 'name' => $request->name, 'slug' => $request->slug, 'parent_id' =>$request->parent_id ]); return redirect()->back()->with('success', 'Category has been created successfully.'); } } }
Create View for createCategory
views/create-category.blade.php
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <section class="content" style="padding:20px 30%;"> <div class="row"> <div class="col-md-12"> <div class="box box-primary"> <div class="box-header with-border"> <h3 class="box-title">Create Category</h3> </div> <form role="form" method="post"> {{csrf_field()}} <div class="box-body"> <div class="row"> <div class="col-sm-12"> <div class="form-group"> <label>Category name*</label> <input type="text" name="name" class="form-control" placeholder="Category name" value="{{old('name')}}" required /> </div> </div> <div class="col-sm-12"> <div class="form-group"> <label>Category Slug*</label> <input type="text" name="slug" class="form-control" placeholder="Category name" value="{{old('slug')}}" required /> </div> </div> <div class="col-sm-12"> <div class="form-group"> <label>Select parent category*</label> <select type="text" name="parent_id" class="form-control"> <option value="">None</option> @if($categories) @foreach($categories as $category) <?php $dash=''; ?> <option value="{{$category->id}}">{{$category->name}}</option> @if(count($category->subcategory)) @include('subCategoryList-option',['subcategories' => $category->subcategory]) @endif @endforeach @endif </select> </div> </div> </div> </div> <div class="box-footer"> <button type="submit" class="btn btn-primary">Create</button> </div> </form> @if ($errors->any()) <div> @foreach ($errors->all() as $error) <li class="alert alert-danger">{{ $error }}</li> @endforeach </div> @endif @if(\Session::has('error')) <div> <li class="alert alert-danger">{!! \Session::get('error') !!}</li> </div> @endif @if(\Session::has('success')) <div> <li class="alert alert-success">{!! \Session::get('success') !!}</li> </div> @endif </div> </div> </div> </section>
views/subcategoryList-option.blade.php
<?php $dash.='-- '; ?> @foreach($subcategories as $subcategory) <option value="{{$subcategory->id}}">{{$dash}}{{$subcategory->name}}</option> @if(count($subcategory->subcategory)) @include('subCategoryList-option',['subcategories' => $subcategory->subcategory]) @endif @endforeach
I hope it will assist you…