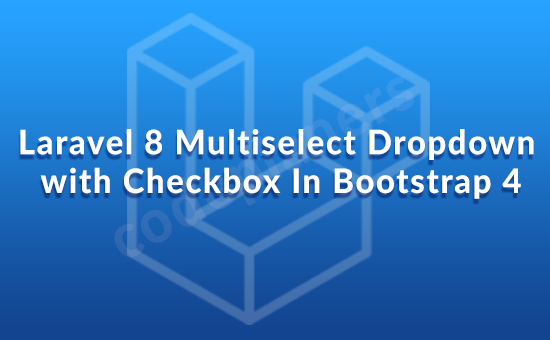
Hi Dev,
Today, i we will show you laravel 8 multiselect dropdown with checkbox in bootstrap 4. This article will give you simple example of laravel 8 multiselect dropdown with checkbox in bootstrap 4. you will learn laravel 8 multiselect dropdown with checkbox in bootstrap 4.
In this artical i will show you laravel 8 multiselect dropdown with checkbox in bootstrap 4. When we make a blog website, we need the multiselect dropdown function. We can use this example in Laravel 6, Laravel 7, Laravel 8, all. So let’s follow few step to create example of laravel 8 multiselect dropdown with checkbox in bootstrap 4.
Preview:
Step 1:- Install Laravel
First of, open your terminal and install new Laravel:
composer create-project --prefer-dist laravel/laravel multiselect
Step 2:- Connect Database .env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=databse DB_USERNAME=root DB_PASSWORD=
Step 3:- Create Modal and Migration
In this step, we need to create category table and model
php artisan make:model Category -m
database\migrations\create_categories_table.php
public function up() { Schema::create('categories', function (Blueprint $table) { $table->id(); $table->string('name'); $table->timestamps(); }); }
app\Models\Category.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Category extends Model { protected $fillable = [ 'name' ]; }
run following command
php artisan migrate
Step 4:- Add Routes
use App\Http\Controllers\CategoryController; Route::get('cat', [CategoryController::class, 'index']); Route::post('store', [CategoryController::class, 'store']);
Step 5:- Create Controllers
run following command and create controllers
php artisan make:controller CategoryController
app/http/controller\CategoryController.php
<?php namespace App\Http\Controllers; use App\Models\Category; use Illuminate\Http\Request; use Illuminate\Support\Facades\Route; class CategoryController extends Controller { public function index() { return view('index'); } public function store(Request $request) { $input = $request->all(); $data = []; $data['name'] = json_encode($input['name']); Category::create($data); return response()->json(['success'=>'Recoreds inserted']); } }
Step 6:- Create Blade Views
In this step, create one blade views file. resources/views folder and create the blade view file:
Create file index.blade.php and add code:
<!DOCTYPE html> <html> <head> <title>Laravel 8 Multiselect Dropdown with Checkbox In Bootstrap 4</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-multiselect/0.9.13/js/bootstrap-multiselect.js"></script> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-multiselect/0.9.13/css/bootstrap-multiselect.css" /> <meta name="csrf-token" content="{{ csrf_token() }}"> </head> <body style="background: #8ab4e8;"> <div class="container" > <h2 align="center">Laravel 8 Multiselect Dropdown with Checkbox In Bootstrap 4</h2> <form method="post" id="category_form"> <div class="form_select"> <label>Select</label> <select id="category" name="name[]" multiple class="form-select" > <option value="Codeigniter">Codeigniter</option> <option value="CakePHP">CakePHP</option> <option value="Laravel">Laravel</option> <option value="YII">YII</option> <option value="Zend">Zend</option> <option value="Symfony">Symfony</option> <option value="Phalcon">Phalcon</option> <option value="Slim">Slim</option> </select> </div> <div class="form-group"> <input type="submit" class="btn btn-primary" name="submit" value="Submit" /> </div> </form> </div> <style> form#category_form { background: #f2f2f2; padding: 50px; width: 50%; margin: 0 auto; } .form-group { text-align: right; } button.multiselect.dropdown-toggle.btn.btn-default { border: 1px solid; margin: 0 0 10px; display: flex; align-items: center; justify-content: space-between; } </style> </body> <script> $(document).ready(function(){ $('#category').multiselect({ nonSelectedText: 'Select category', enableFiltering: true, enableCaseInsensitiveFiltering: true, buttonWidth:'400px' }); $('#category_form').on('submit', function(event){ event.preventDefault(); var form_data = $(this).serialize(); $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); $.ajax({ url:"{{ url('store') }}", method:"POST", data:form_data, success:function(data) { $('#category option:selected').each(function(){ $(this).prop('selected', false); }); $('#category').multiselect('refresh'); alert(data['success']); } }); }); }); </script> </html>
Step 7:- Run Development Server
php artisan serve
http://127.0.0.1:8000/cat