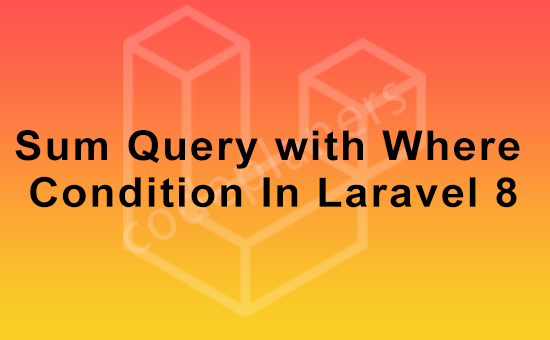
Hi Dev,
Today, i we will show you sum query with where condition in laravel 8. This article will give you simple example of sum query with where condition in laravel 8. you will sum query with where condition in laravel 8. In this article, we will implement a sum query with where condition in laravel 8.
So let’s follow few step to create example of sum query with where condition in laravel 8.
Example 1: using having()
<?php namespace App\Http\Controllers; use App\Models\User; use DB; class UserController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $users = UserPayment::select("*", DB::raw('SUM(amount) as total')) ->groupBy("user_id") ->having('total', '>', 50) ->get(); dd($users); } }
Output:
Array ( [0] => Array ( [id] => 1 [user_id] => 1 [amount] => 56 [payment_date] => 2022-01-04 [status] => 1 [created_at] => [updated_at] => [total] => 56 ) [1] => Array ( [id] => 2 [user_id] => 2 [amount] => 45 [payment_date] => 2022-01-07 [status] => 0 [created_at] => [updated_at] => [total] => 55 ) )
Example 2: using havingRaw()
<?php namespace App\Http\Controllers; use App\Models\User; use DB; class UserController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $users = UserPayment::select("*", DB::raw('SUM(amount) as total')) ->groupBy("user_id") ->havingRaw('total > 50') ->get(); dd($users); } }
Output:
Array ( [0] => Array ( [id] => 1 [user_id] => 1 [amount] => 58 [payment_date] => 2022-01-04 [status] => 1 [created_at] => [updated_at] => [total] => 56 ) [1] => Array ( [id] => 2 [user_id] => 2 [amount] => 45 [payment_date] => 2022-01-07 [status] => 0 [created_at] => [updated_at] => [total] => 55 ) )
I hope it will assist you…