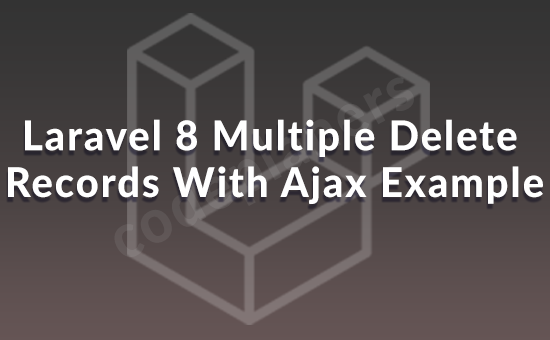
Hi Dev,
Today, i we will show you laravel 8 multiple delete records with ajax example. This article will give you simple example of laravel 8 multiple delete records with ajax example. you will learn laravel 8 multiple delete records with ajax example.
In this artical i will show you laravel 8 multiple delete records with ajax example. We can use this example in Laravel 6, Laravel 7, Laravel 8, all. So let’s follow few step to create example of laravel 8 multiple delete records with ajax example.
Preview:
Step 1:- Install Laravel
First of, open your terminal and install new Laravel:
composer create-project --prefer-dist laravel/laravel multipledeleterecords
Step 2:- Connect Database .env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=databse DB_USERNAME=root DB_PASSWORD=
Step 3:- Create Modal and Migration
In this step, we need to create category table and model
php artisan make:model Category -m
database\migrations\create_categories_table.php
public function up() { Schema::create('categories', function (Blueprint $table) { $table->id(); $table->string('name'); $table->text('description'); $table->timestamps(); }); }
app\Models\Category.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Category extends Model { protected $fillable = [ 'name','description' ]; }
run following command
php artisan migrate
Step 4:- Add Routes
use App\Http\Controllers\CategoryController; Route::get('cat', [CategoryController::class, 'index']); Route::get('store', [CategoryController::class, 'show']); Route::post('store', [CategoryController::class, 'store']); Route::get('category/{id}', [CategoryController::class, 'destroy'])->name('category.destroy'); Route::delete('delete-multiple-category', [CategoryController::class, 'deleteMultiple'])->name('category.multiple-delete');
Step 5:- Create Controllers
run following command and create controllers
php artisan make:controller CategoryController
app/http/controller\CategoryController.php
<?php namespace App\Http\Controllers; use App\Models\Category; use Illuminate\Http\Request; use Illuminate\Support\Facades\Route; use Illuminate\Support\Facades\Validator; class CategoryController extends Controller { public function index(Request $request) { $data['categories'] = Category::get(); return view('index', $data); } public function show(Request $request) { return view('store'); } public function store(Request $request) { $this->validate($request, [ 'name' => 'required', ]); $category = new Category; $category->name = $request['name']; $category->description = $request['description']; $category->save(); return redirect('/cat'); } public function destroy(Request $request,$id) { $category=Category::find($id); $category->delete(); return back()->with('success','Category deleted successfully'); } public function deleteMultiple(Request $request) { $ids = $request->ids; Category::whereIn('id',explode(",",$ids))->delete(); return response()->json(['status'=>true,'message'=>"Category deleted successfully."]); } }
Step 6:- Create Blade Views
In this step, create one blade views file. resources/views folder and create the blade view file:
Create file index.blade.php and add code:
<!DOCTYPE html> <html> <head> <title>Laravel 8 Multiple Delete Records With Ajax Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/css/bootstrap.min.css" > <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/js/bootstrap.bundle.min.js" ></script> <meta name="csrf-token" content="{{ csrf_token() }}"> </head> <body> <div class="container"> <h3>Laravel 8 Multiple Delete Records With Ajax Example</h3> @if ($message = Session::get('success')) <div class="alert alert-success"> <p>{{ $message }}</p> </div> @endif <button style="margin: 5px;" class="btn btn-danger btn-xs delete-all" data-url="">Delete All</button> <a class="btn btn-primary btn-xs float-right" href="{{ url('store')}}">Add Category</a> <table class="table table-bordered"> <tr> <th><input type="checkbox" id="check_all"></th> <th>S.No.</th> <th>Category Name</th> <th>Category Details</th> <th width="100px">Action</th> </tr> @if($categories->count()) @foreach($categories as $key => $category) <tr id="tr_{{$category->id}}"> <td><input type="checkbox" class="checkbox" data-id="{{$category->id}}"></td> <td>{{ ++$key }}</td> <td>{{ $category->name }}</td> <td>{{ $category->description }}</td> <td> <form method="delete" action="{{ url('category').'/'.$category->id}}" style="display:inline;" > <button class="btn btn-danger btn-xs" data-toggle="confirmation" type="submit">Delete</button> </form> </td> </tr> @endforeach @endif </table> </div> </body> <script type="text/javascript"> $(document).ready(function () { $('#check_all').on('click', function(e) { if($(this).is(':checked',true)) { $(".checkbox").prop('checked', true); } else { $(".checkbox").prop('checked',false); } }); $('.checkbox').on('click',function(){ if($('.checkbox:checked').length == $('.checkbox').length){ $('#check_all').prop('checked',true); }else{ $('#check_all').prop('checked',false); } }); $('.delete-all').on('click', function(e) { var idsArr = []; $(".checkbox:checked").each(function() { idsArr.push($(this).attr('data-id')); }); if(idsArr.length <=0) { alert("Please select atleast one record to delete."); } else { if(confirm("Are you sure, you want to delete the selected categories?")){ var strIds = idsArr.join(","); $.ajax({ url: "{{ route('category.multiple-delete') }}", type: 'DELETE', headers: {'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')}, data: 'ids='+strIds, success: function (data) { if (data['status']==true) { $(".checkbox:checked").each(function() { $(this).parents("tr").remove(); }); alert(data['message']); } else { alert('Whoops Something went wrong!!'); } }, error: function (data) { alert(data.responseText); } }); } } }); $('[data-toggle=confirmation]').confirmation({ rootSelector: '[data-toggle=confirmation]', onConfirm: function (event, element) { element.closest('form').submit(); } }); }); </script> </html>
Create file store.blade.php and add code:
<!DOCTYPE html> <html> <head> <title>Laravel 8 Multiple Delete Records With Ajax Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/css/bootstrap.min.css" > <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/js/bootstrap.bundle.min.js" ></script> <meta name="csrf-token" content="{{ csrf_token() }}"> </head> <body> <div class="container"> <h3>Laravel 8 Multiple Delete Records With Ajax Example</h3> @if ($message = Session::get('success')) <div class="alert alert-success"> <p>{{ $message }}</p> </div> @endif <form method="post" action="{{ url('store') }}" > <input type="hidden" name = "_token" value = "<?php echo csrf_token(); ?>"> <div class="form-row"> <div class="col-md-12 mb-3"> <label for="">Name</label> <input type="text" class="form-control" name="name" placeholder="Name" > </div> <div class="col-md-12 mb-3"> <label for="">Description</label> <textarea class="form-control" name="description" ></textarea> </div> </div> <button class="btn btn-primary" type="submit">Submit</button> </form> </div> </body> </html>
Step 7:- Run Development Server
php artisan serve
http://127.0.0.1:8000/cat