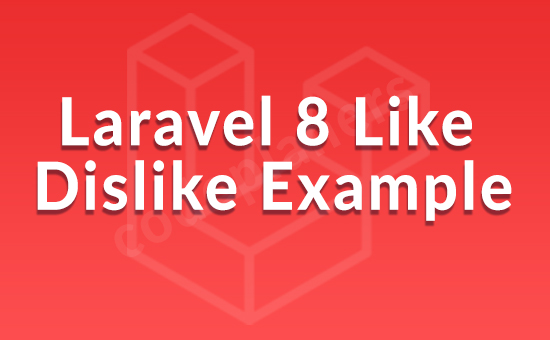
Today, i we will show you Laravel 8 Like Dislike Example. This article will give you simple example of Laravel 8 Like Dislike Example. you will learn Laravel 8 Like Dislike Example. step by step explain Laravel 8 Like Dislike Example.
For making it i will use “overture/laravel-follow” composer package to create follow unfollow system in Laravel.
Step 1 :- Install Laravel
composer create-project --prefer-dist laravel/laravel blog
Connect Database .env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=databse DB_USERNAME=root DB_PASSWORD=
Step 2 :- Install overtrue/laravel-follow Package
composer require overtrue/laravel-follow -vvv
Now open config/app.php file and add service provider and aliase.
config/app.php
'providers' => [ Overtrue\LaravelFollow\FollowServiceProvider::class, ],
To publish the migrations file run bellow command.
php artisan vendor:publish --provider='Overtrue\LaravelFollow\FollowServiceProvider' --tag="migrations"
As optional if you want to modify the default configuration, you can publish the configuration file.
php artisan vendor:publish --provider="Overtrue\LaravelFollow\FollowServiceProvider" --tag="config"
Then just migrate it by using following command
php artisan migrate
Step 3 :- Create Authentication
php artisan make:auth
Step 4 :- Create Posts
php artisan make:migration create_posts_table
database/migrations/CreatePostsTable.php
use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreatePostsTable extends Migration { public function up() { Schema::create('posts', function (Blueprint $table) { $table->increments('id'); $table->string('title'); $table->timestamps(); }); } public function down() { Schema::dropIfExists('posts'); } }
php artisan migrate
After this we need to create model for posts table by following path.
App/Post.php
namespace App; use Overtrue\LaravelFollow\Traits\CanBeLiked; use Illuminate\Database\Eloquent\Model; class Post extends Model { use CanBeLiked; protected $fillable = ['title']; }
Now we require to create some dummy posts data on database table so create laravel seed using bellow command:
php artisan make:seeder CreateDummyPost
update CreateDummyPost seeds like as bellow:
database/seeds/CreateDummyPost.php
use Illuminate\Database\Seeder; use App\Post; class CreateDummyPost extends Seeder { public function run() { $posts = ['codechief.org', 'wordpress.org', 'laramust.com']; foreach ($posts as $key => $value) { Post::create(['title'=>$value]); } } }
Run seeder using this command.
php artisan db:seed --class=CreateDummyPost
Step 5 :- Update User Model
App/User.php
namespace App; use Overtrue\LaravelFollow\Traits\CanLike; use Illuminate\Notifications\Notifiable; use Illuminate\Foundation\Auth\User as Authenticatable; class User extends Authenticatable { use CanLike, Notifiable ; //Notice we used CanLike trait protected $fillable = [ 'name', 'email', 'password', ]; protected $hidden = [ 'password', 'remember_token', ]; }
Step 6 :- Create Routes
routes/web.php
Route::get('/home', 'HomeController@index')->name('home'); Route::get('posts', 'HomeController@posts')->name('posts'); Route::post('like', 'HomeController@LikePost')->name('like');
Step 7 :- Add Controller
app/Http/HomeController.php
namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Post; use App\User; class HomeController extends Controller { public function __construct() { $this->middleware('auth'); } public function index() { return view('home'); } public function posts() { $posts = Post::get(); return view('posts', compact('posts')); } public function LikePost(Request $request){ $post = Post::find($request->id); $response = auth()->user()->toggleLike($post); return response()->json(['success'=>$response]); } }
Step 8 :- Add Blade file
resources/views/posts.blade.php
@extends('layouts.app') @section('content') <link rel="stylesheet" type="text/css" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <meta name="csrf-token" content="{{ csrf_token() }}" /> <link href="{{ asset('css/custom.css') }}" rel="stylesheet"> <div class="container"> <div class="row justify-content-center"> <div class="col-md-12"> <div class="card"> <div class="card-header">Posts</div> <div class="card-body"> @if($posts->count()) @foreach($posts as $post) <article class="col-xs-12 col-sm-6 col-md-3"> <div class="panel panel-info" data-id="{{ $post->id }}"> <div class="panel-body"> <a href="https://www.codechief.org/user/img/user.jpg" title="Nature Portfolio" data-title="Amazing Nature" data-footer="The beauty of nature" data-type="image" data-toggle="lightbox"> <img src="https://www.codechief.org/user/img/user.jpg" style="height: 50px; width: 50px; border-radius: 50%;"> <span class="overlay"><i class="fa fa-search"></i></span> </a> </div> <div class="panel-footer"> <h4><a href="#" title="Nature Portfolio">{{ $post->title }}</a></h4> <span class="pull-right"> <span class="like-btn"> <i id="like{{$post->id}}" class="glyphicon glyphicon-thumbs-up {{ auth()->user()->hasLiked($post) ? 'like-post' : '' }}"></i> <div id="like{{$post->id}}-bs3">{{ $post->likers()->get()->count() }}</div> </span> </span> </div> </div> </article> @endforeach @endif </div> </div> </div> </div> </div> <script type="text/javascript"> $(document).ready(function() { $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); $('i.glyphicon-thumbs-up, i.glyphicon-thumbs-down').click(function(){ var id = $(this).parents(".panel").data('id'); var c = $('#'+this.id+'-bs3').html(); var cObjId = this.id; var cObj = $(this); $.ajax({ type:'POST', url:'/like', data:{id:id}, success:function(data){ if(jQuery.isEmptyObject(data.success.attached)){ $('#'+cObjId+'-bs3').html(parseInt(c)-1); $(cObj).removeClass("like-post"); }else{ $('#'+cObjId+'-bs3').html(parseInt(c)+1); $(cObj).addClass("like-post"); } } }); }); $(document).delegate('*[data-toggle="lightbox"]', 'click', function(event) { event.preventDefault(); $(this).ekkoLightbox(); }); }); </script> @endsection