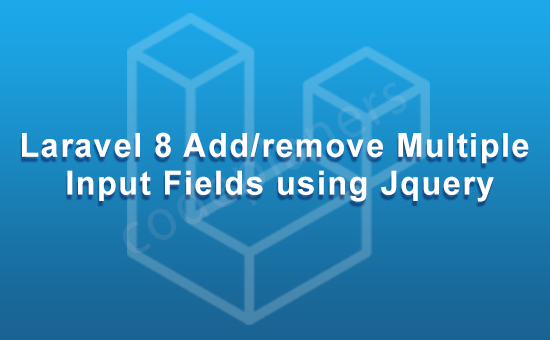
Hi,
Today, i we will show you laravel 8 add/remove multiple input fields using jquery. This article will give you simple example of laravel 8 add/remove multiple input fields using jquery. you will learn laravel 8 add/remove multiple input fields using jquery. So let’s follow few step to create example of laravel 8 add/remove multiple input fields using jquery.
Step 1: Install Laravel
composer create-project --prefer-dist laravel/laravel blog
Step 2: Database Configuration
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=database name DB_USERNAME=database username DB_PASSWORD=database password
Step 3: Create Table and Model
open a command and run the following command:
php artisan make:model Contact -m
app/models/Contact.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Contact extends Model { use HasFactory; protected $fillable = [ 'title', 'description' ]; }
database/migrations/create_contact_table.php
public function up() { Schema::create('todos', function (Blueprint $table) { $table->id(); $table->string('title'); $table->text('description'); $table->timestamps(); }); }
php artisan migrate
Step 4: Create Route
use App\Http\Controllers\ContactController; Route::get('input-fields', [ContactController::class, 'index']); Route::post('input-fields', [ContactController::class, 'store']);
Step 5: Create Controller
App\Http\Controllers\ContactController
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Contact; use Illuminate\Support\Facades\Validator; class ContactControllerextends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { return view("input-fields"); } public function store(Request $request) { $request->validate([ 'moreFields.*.title' => 'required', 'moreFields.*.description' => 'required', ]); foreach ($request->moreFields as $key => $value) { Todo::create($value); } return back()->with('success', 'Todos Has Been Created Successfully.'); } }
Step 6: Create Blade
<!DOCTYPE html> <html> <head> <title>Laravel 8 Add/remove Multiple Input Fields using Jquery</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.1/css/bootstrap.min.css"> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.1/js/bootstrap.min.js"></script> <meta name="csrf-token" content="{{ csrf_token() }}"> </head> <body> <div class="container"> <div class="card mt-3"> <div class="card-header"><h2>Laravel 8 Add/remove Multiple Input Fields using Jquery</h2></div> <div class="card-body"> <form action="{{ url('input-fields') }}" method="POST"> @csrf @if ($errors->any()) <div class="alert alert-danger"> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif @if (Session::has('success')) <div class="alert alert-success text-center"> <a href="#" class="close" data-dismiss="alert" aria-label="close">×</a> <p>{{ Session::get('success') }}</p> </div> @endif <table class="table table-bordered" id="dynamicAddRemove"> <tr> <th>Title</th> <th>Description</th> <th>Action</th> </tr> <tr> <td><input type="text" name="moreFields[0][title]" placeholder="Enter title" class="form-control" /></td> <td><input type="text" name="moreFields[0][description]" placeholder="Enter description" class="form-control" /></td> <td><button type="button" name="add" id="add-btn" class="btn btn-success">Add More</button></td> </tr> </table> <button type="submit" class="btn btn-success">Save</button> </form> </div> </div> </div> <script type="text/javascript"> var i = 0; $("#add-btn").click(function(){ ++i; $("#dynamicAddRemove").append('<tr><td><input type="text" name="moreFields['+i+'][title]" placeholder="Enter title" class="form-control" /></td><td><input type="text" name="moreFields['+i+'][description]" placeholder="Enter description" class="form-control" /></td><td><button type="button" class="btn btn-danger remove-tr">Remove</button></td></tr>'); }); $(document).on('click', '.remove-tr', function(){ $(this).parents('tr').remove(); }); </script> </body> </html>
Step 7: Run Server
php artisan serve