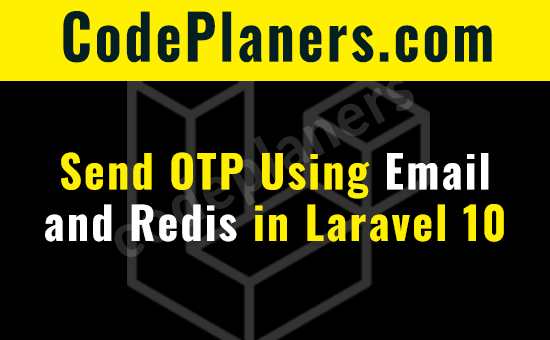
Hi Dev,
Today, we will show you send OTP using Email and Redis in Laravel 10. This article will give you simple example of send OTP using Email and Redis in Laravel 10. Let’s discuss send OTP using Email and Redis in Laravel 10. In this article, we will implement a send OTP using Email and Redis in Laravel 10.
So let’s follow few step to create example of send OTP using Email and Redis in Laravel 10.
Step 1: Install Laravel 10
composer create-project laravel/laravel send-otp
Step 2: Configure Mail and Redis
.env file with the required configurations for mail and Redis.
MAIL_MAILER=smtp MAIL_HOST=your mail host MAIL_PORT=your mail port MAIL_USERNAME=your mail username MAIL_PASSWORD=your mail password MAIL_ENCRYPTION=tls
REDIS_HOST=127.0.0.1 REDIS_PASSWORD=your redis password REDIS_PORT=6379
Replace the placeholders with your actual mail and redis configuration.
Step 3: Create a Controller
php artisan make:controller OtpController
app/Http/Controllers/OtpController.php
<?php namespace App\Http\Controllers; use Illuminate\Support\Facades\Mail; use Illuminate\Support\Facades\Redis; use App\Mail\OtpMail; use Illuminate\Http\Request; class OtpController extends Controller { /** * write code for. * * @param \Illuminate\Http\Request * @return \Illuminate\Http\Response * @author <> */ public function sendOtp(Request $request) { $email = $request->input(‘email’); $otp = rand(100000, 999999); Redis::setex(“otp:$email”, 300, $otp); Mail::to($email)->send(new OtpMail($otp)); return response()->json([‘message’ => ‘OTP sent successfully’]); } }
This controller will generate a random 6-digit OTP, store it in Redis with a 5-minute expiration and later ring the given email address.
Step 4: Create a Mailable
OtpMail.php located in the app/Mail directory
<?php namespace App\Mail; use Illuminate\Bus\Queueable; use Illuminate\Mail\Mailable; use Illuminate\Queue\SerializesModels; use Illuminate\Contracts\Queue\ShouldQueue; class OtpMail extends Mailable { use Queueable, SerializesModels; public $otp; /** * Create a new message instance. * * @return void */ public function __construct($otp) { $this->otp = $otp; } /** * Build the message. * * @return $this */ public function build() { return $this->view('emails.otp'); } }
Step 5: Create an Email View
resources/views/emails/otp.blade.php
<!DOCTYPE html> <html> <head> <title>One-Time Password</title> </head> <body> <h1>Your OTP is: {{ $otp }}</h1> </body> </html>
Step 6: Update Routes
web.php
use App\Http\Controllers\OtpController; Route::post(‘/send-otp’, [OtpController::class, ‘sendOtp’]);
Step 7: Run
php artisan serve
I hope it will assist you…